How do I retrieve the logged in Google account on android phones?
Solution 1
Something like this should work:
AccountManager manager = (AccountManager) getSystemService(ACCOUNT_SERVICE);
Account[] list = manager.getAccounts();
String gmail = null;
for(Account account: list)
{
if(account.type.equalsIgnoreCase("com.google"))
{
gmail = account.name;
break;
}
}
And you will need the following permission in your manifest:
<uses-permission android:name="android.permission.GET_ACCOUNTS"></uses-permission>
Remember to 'Requesting Permissions at Run Time' if you support Android 6 and later https://developer.android.com/training/permissions/requesting.html
I wrote this from memory so it may need a little tweaking. Apparently it's possible to register now without an email address, so maybe do some regexing on the data to ensure it's actually an email address (ensure it contains @gmail or @googlemail)
Solution 2
I have try below scope to get email address and username
Get Google account in your mobile
public String getMailId() {
String strGmail = null;
try {
Account[] accounts = AccountManager.get(this).getAccounts();
Log.e("PIKLOG", "Size: " + accounts.length);
for (Account account : accounts) {
String possibleEmail = account.name;
String type = account.type;
if (type.equals("com.google")) {
strGmail = possibleEmail;
Log.e("PIKLOG", "Emails: " + strGmail);
break;
}
}
} catch (Exception e) {
e.printStackTrace();
strGmail = null;
}
return strGmail;
}
Get Google accounts user name in your mobile
public String getUsername() {
List<String> possibleEmails = null;
try {
AccountManager manager = AccountManager.get(this);
Account[] accounts = manager.getAccountsByType("com.google");
possibleEmails = new LinkedList<>();
for (Account account : accounts) {
// TODO: Check possibleEmail against an email regex or treat
// account.name as an email address only for certain account.type
// values.
possibleEmails.add(account.name);
}
} catch (Exception e) {
e.printStackTrace();
if (possibleEmails != null) {
possibleEmails.clear();
}
}
if (possibleEmails != null) {
if (!possibleEmails.isEmpty() && possibleEmails.get(0) != null) {
String email = possibleEmails.get(0);
String[] parts = email.split("@");
if (parts.length > 0 && parts[0] != null) {
return parts[0];
} else {
return null;
}
} else {
return null;
}
} else {
return null;
}
}
declare permissions to your mainfest file.
<uses-permission android:name="android.permission.GET_ACCOUNTS" />
Related videos on Youtube
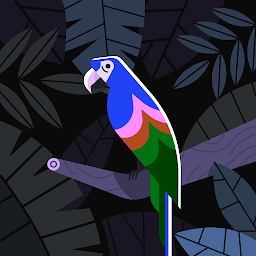
Samuel Overduib
Updated on July 09, 2022Comments
-
Samuel Overduib almost 2 years
I am developing an Android application and I need to retrieve the Google account used on the phone. I want to do this for the C2DM, but I don't want to ask the user to enter in his/her Google email account if they are already logged in. Is there any way to do it?
-
Luís Oliveira over 13 yearsAnyone aware of similar solutions compatible with Android 1.6?
-
Luís Oliveira over 13 yearsFound an answer here: stackoverflow.com/questions/3360926/…
-
SHRISH M over 12 yearsA phone can have more then one gmail account registered, you should enable the user to choose one of this
-
Andrew Wyld about 12 yearsJust to clarify, is it possible for two or more members of
list
BOTH to have the type "com.google"? If so, how do you distinguish the logged in one? -
Teario about 12 yearsIt is possible, and in that case they are both logged in. I have my personal Google account and the Google account that my employer gave me for work on my phone. I get email alerts from both, on the Play store I have sections for both accounts in my 'Installed' list and the Calendar app shows entries from both accounts. In such a situation you'd most likely be after the device's main account (the one entered first, that can't be unlinked without a factory reset). Unfortunately I don't know of a way to tell which one is the main account.
-
SimpleCoder over 5 yearsdoes it still work ? I am trying same but not working ?