How do I send an email from a WinRT/Windows Store application?
Solution 1
The correct way would be to use Sharing. Your app should create an HTML document or Text and share it. The user would select Mail from the Share charm and the HTML/Text would become the body of the email.
See here for more info...
http://msdn.microsoft.com/en-us/library/windows/apps/xaml/hh973055.aspx
Solution 2
You can try with
var mailto = new Uri("mailto:[email protected]&subject=The subject of an email&body=Hello from a Windows 8 Metro app.");
await Windows.System.Launcher.LaunchUriAsync(mailto);
Solution 3
This is the correct syntax to use for a mailto: link (unlike the other examples above with a mailto: which are incorrect..)
var mailto = new Uri("mailto:[email protected]?subject=" + subject + "&body=" + body);
await Launcher.LaunchUriAsync(mailto);
The problem with the mailto: method is if the user has no client program associated with mailto: nothing will happen.
The most reliable method to use is a web service or WCF service of some sort. Using the Share Charm while considered the 'correct' way on Windows 8, is not neccessarily the best as the user may still have no email client installed, for example if they rely on gmail.com for their email.
Solution 4
If you are developping a Universal WinRT Windows Phone application, you could use the "Windows.ApplicationModel.Email.EmailMessage" namespace as the "Microsoft.Phone.Tasks.EmailComposeTask" namespace doesn't work on WinRT application.
Then, uses this code to create and launch a new email.
// Create your new email message.
var em = new EmailMessage() ;
// Add as much EmailRecipient in it as you need using the following method.
em.To.Add(new EmailRecipient("[email protected]"));
em.Subject = "Your Subject...";
em.Body = "Your email body...";
// You can add an attachment that way.
//em.Attachments.Add(new EmailAttachment(...);
// Show the email composer.
await EmailManager.ShowComposeNewEmailAsync(em);
I hope it will solve your (or other developers) problem.
Regards.
Solution 5
It's always possible to connect to an SMTP server and issue commands like HELO, MAIL, RCPT, etc. Of course you'll need an SMTP server to connect to. I use this on our corporate intranet to send emails.
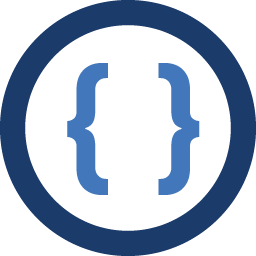
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
I am developing a Windows Store Application (Windows 8).
I have a need to send emails based on data and address stored in the application data and without the need of the user to type it the data or the address.
What would be the right/easy way to implement it?
EitanB