How do I use a StreamProvider from a StateNotifierProvider?
218
The simplest way to accomplish this is to accept a Reader
as a parameter to your StateNotifier.
For example:
class CartRiverpod extends StateNotifier<List<CartItemModel>> {
CartRiverpod(this._read, [List<CartItemModel> products]) : super(products ?? []) {
// use _read anywhere in your StateNotifier to access any providers.
// e.g. _read(productListStreamProvider);
}
final Reader _read;
void add(ProductModel product) {
state = [...state, new CartItemModel(product: product)];
print("added");
}
void remove(String id) {
state = state.where((product) => product.id != id).toList();
}
}
final cartRiverpodProvider = StateNotifierProvider<CartRiverpod>((ref) => CartRiverpod(ref.read, []));
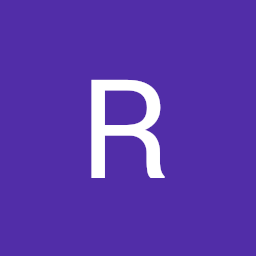
Author by
Rahul Denmoto
Updated on December 27, 2022Comments
-
Rahul Denmoto over 1 year
I am try to use a StreamProvider from a StateNotifierProvider.
Here is my StreamProvider, which works fine so far.
final productListStreamProvider = StreamProvider.autoDispose<List<ProductModel>>((ref) { CollectionReference ref = FirebaseFirestore.instance.collection('products'); return ref.snapshots().map((snapshot) { final list = snapshot.docs .map((document) => ProductModel.fromSnapshot(document)) .toList(); return list; }); });
Now I am trying to populate my shopping cart to have all the products in it from scratch.
final cartRiverpodProvider = StateNotifierProvider((ref) => new CartRiverpod(ref.watch(productListStreamProvider));
This is my CartRiverPod StateNotifier
class CartRiverpod extends StateNotifier<List<CartItemModel>> { CartRiverpod([List<CartItemModel> products]) : super(products ?? []); void add(ProductModel product) { state = [...state, new CartItemModel(product:product)]; print ("added"); } void remove(String id) { state = state.where((product) => product.id != id).toList(); } }
-
Rahul Denmoto about 3 yearsAlex, thank you so much! That's exactly what I was looking for.
-
Alex Hartford about 3 years@RahulDenmoto You're welcome. Please mark the answer as accepted to aid future readers. Glad it worked out for you!