How do I use a variable from an application.yml file in my normal code?
17,647
Solution 1
You can use this:
@Value("${your.path.yml.string}")
private String x;
YML:
your:
path:
yml:
string: hello
x will be "hello"
Solution 2
- You need to use Spring Expression Language which says we should write it as
@Value("${spring.application.name}")
private String appName;
- For Default value if key is not present in yaml/yml or properties file
@Value("${spring.application.name: defaultValue}")
private String appName;
- The last way you can fetch value is using environment object
@Autowired
private Environment environment;
String appName = environment.get("spring.application.name");
Solution 3
You can add @Value
annotation to any field in your beans.
@Value("$(path.to.your.variable)")
String myString;
Works with constructors as well.
public MyClass(@Value("$(path.to.your.variable)") String myString) {
Solution 4
You can use @Value
on fields or parameters to assign the property to some variable.
Property example:
@Value("${indicator}")
private String indicator
Parameter example:
private void someMethod(@Value("${indicator}") String indicator) {
...
}
Then you can use indicator as you want.
Note: the class where you use @Value
should be a Spring Component
Related videos on Youtube
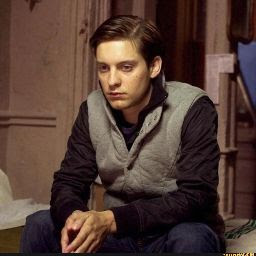
Author by
nolanite1
Updated on June 04, 2022Comments
-
nolanite1 almost 2 years
For example, let's say that in my yml file I had a variable called indicator. And based on what the indicator variable's value was I want the code to do something different. How would I access the yml variable in the regular code and use it accordingly?
-
Arjun Kalidas over 2 yearsRefer this link: baeldung.com/spring-yaml this helped me understand this better.
-
-
chrylis -cautiouslyoptimistic- almost 4 yearsThis entire functionality is one of the core things Spring provides in the first place.
-
Rishabh Sharma almost 4 yearsIt is not mentioned anywhere in the question that spring is required (inspite of the tag). I guess - "To a man with a hammer, everything looks like a nail" :)
-
nolanite1 almost 4 yearswhat would the path be like? just the path to the yaml file where the variable is?
-
chrylis -cautiouslyoptimistic- almost 4 yearsIt's tagged
spring-boot
and he mentionsapplication.yml
by name. -
nolanite1 almost 4 yearsoh ok. when i tried that i got an error saying Unsatisfied dependency expressed through field 'sampleService'; nested exception is org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'sampleServiceImpl': Injection of autowired dependencies failed; nested exception is java.lang.IllegalArgumentException: Could not resolve placeholder 'indicator.operation' in value "${indicator.string}
-
luisfa19 almost 4 yearsuse @Component in the class.
-
nolanite1 almost 4 yearswait the class i'm using it in or do i have to create a class for the variable?
-
luisfa19 almost 4 yearscould be in the class you are using it