How do I use an .ashx handler with an asp:Image object?
Solution 1
You are printing out the path to the image instead of the actual image contents. Use
context.Response.WriteFile(context.Server.MapPath(imagePath));
method instead.
Make sure you restrict the path to some safe location. Otherwise, you might introduce security vulnerabilities as users would be able to see contents of any file on the server.
Solution 2
This code help to Viewing image by File name From Specified Path.
ex. http://onlineshoping.somee.com/Fimageview.ashx?FImageName=FrozenToront.Jpg
And You may use .ashx in your ASPX html body.
Example:
<image src="/Timageview.ashx?TImageName=FrozenToront.Jpg"
width="100" height="75" border="1"/>
<%@
WebHandler Language="C#"
Class="Fimageview"
%>
/// <summary>
/// Created By Rajib Chowdhury Mob. 01766-306306; Web: http://www.rajibs.tk
/// Email: [email protected]
/// FB: https://www.facebook.com/fencefunny
///
/// Complete This Page Coding On Fabruary 12, 2014
/// Programing C# By Visual Studio 2013 For Web
/// Dot Net Version 4.5
/// Database Virsion MSSQL Server 2005
/// </summary>
using System;
using System.IO;
using System.Web;
public class Fimageview : IHttpHandler {
public void ProcessRequest(HttpContext context)
{
HttpResponse r = context.Response;
r.ContentType = "image/png";
string file = context.Request.QueryString["FImageName"];
if (string.IsNullOrEmpty(file))
{
context.Response.Redirect("~/default");//If RequestQueryString Null Or Empty
}
try
{
if (file == "")
{
context.Response.Redirect("~/Error/PageNotFound");
}
else
{
r.WriteFile("/Catalog/Images/" + file); //Image Path If File Name Found
}
}
catch (Exception)
{
context.Response.ContentType = "image/png";
r.WriteFile("/yourImagePath/NoImage.png");//Image Path If File Name Not Found
}
}
public bool IsReusable
{
get
{
return false;
}
}
}
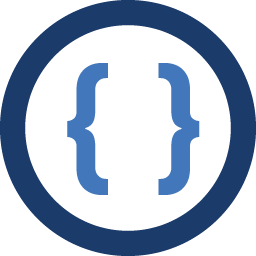
Admin
Updated on July 18, 2022Comments
-
Admin almost 2 years
I have an ashx handler:
<%@ WebHandler Language="C#" Class="Thumbnail" %> using System; using System.Web; public class Thumbnail : IHttpHandler { public void ProcessRequest(HttpContext context) { string imagePath = context.Request.QueryString["image"]; // split the string on periods and read the last element, this is to ensure we have // the right ContentType if the file is named something like "image1.jpg.png" string[] imageArray = imagePath.Split('.'); if (imageArray.Length <= 1) { throw new HttpException(404, "Invalid photo name."); } else { context.Response.ContentType = "image/" + imageArray[imageArray.Length - 1]; context.Response.Write(imagePath); } } public bool IsReusable { get { return true; } } }
For now all this handler does is get an image and return it. In my aspx page, I have this line:
<asp:Image ID="Image1" runat="server" CssClass="thumbnail" />
And the C# code behind it is:
Image1.ImageUrl = "Thumbnail.ashx?image=../Files/random guid string/test.jpg";
When I view the web page, the images are not showing and the HTML shows exactly what I typed:
<img class="thumbnail" src="Thumbnail.ashx?image=../Files%5Crandom guid string%5Cimages%5Ctest.jpg" style="border-width:0px;" />
Can someone tell me why this isn't working? Unfortunately I only started working with ASP.NET yesterday and I have no idea how it works, so please keep the explanations simple if possible, thanks.
-
Admin almost 15 yearsActually, what I want is only the path because I want to set the
img src
. Later on I plan to have the HttpHandler generate a thumbnail, then return the path to it. -
mmx almost 15 yearsThat's not what a HTTP handler does. The browser just treats the handler as an image file. It can't change the original HTML response of the page.
-
Istiyak Amin over 5 yearsyour example is so nice. it's the best solution i seen ever