How do I use an equivalent to C++ reference parameters in Java?
Solution 1
Simulating reference with wrappers.
One way you can have this behavior somehow simulated is create a generic wrapper.
public class _<E> {
E ref;
public _( E e ){
ref = e;
}
public E g() { return ref; }
public void s( E e ){ this.ref = e; }
public String toString() {
return ref.toString();
}
}
I'm not too convinced about the value of this code, by I couldn't help it, I had to code it :)
So here it is.
The sample usage:
public class Test {
public static void main ( String [] args ) {
_<Integer> iByRef = new _<Integer>( 1 );
addOne( iByRef );
System.out.println( iByRef ); // prints 2
_<String> sByRef = new _<String>( "Hola" );
reverse( sByRef );
System.out.println( sByRef ); // prints aloH
}
// Change the value of ref by adding 1
public static void addOne( _<Integer> ref ) {
int i = ref.g();
ref.s( ++i );
// or
//int i = ref.g();
//ref.s( i + 1 );
}
// Reverse the vale of a string.
public static void reverse( _<String> otherRef ) {
String v = otherRef.g();
String reversed = new StringBuilder( v ).reverse().toString();
otherRef.s( reversed );
}
}
The amusing thing here, is the generic wrapper class name is "_" which is a valid class identifier. So a declaration reads:
For an integer:
_<Integer> iByRef = new _<Integer>( 1 );
For a String:
_<String> sByRef = new _<String>( "Hola" );
For any other class
_<Employee> employee = new _<Employee>( Employee.byId(123) );
The methods "s" and "g" stands for set and get :P
Solution 2
Java has no equivalent of C++ references. The only way to get this to work is to encapsulate the values in another class and swap the values within the class.
Here is a lengthy discussion on the issue: http://www.yoda.arachsys.com/java/passing.html
Solution 3
Java does not have pass-by-reference. You must encapsulate to achieve the desired functionality. Jon Skeet has a brief explanation why pass-by-reference was excluded from Java.
Solution 4
Well, there are a couple of workarounds. You mentioned one yourself. Another one would be:
public void test(int[] values) {
++values[0];
++values[1];
}
I would go with the custom object, though. It’s a much cleaner way. Also, try to re-arrange your problem so that a single method doesn’t need to return two values.
Solution 5
A better question: why are you creating methods with such side-effects?
Generally, this is a strong indication that you should extract the data into a separate class, with public accessors that describe why the operation is taking place.
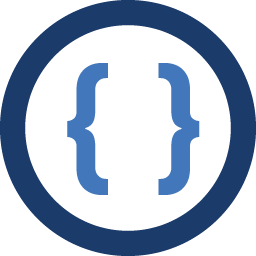
Admin
Updated on June 25, 2022Comments
-
Admin almost 2 years
Suppose I have this in C++:
void test(int &i, int &j) { ++i; ++j; }
The values are altered inside the function and then used outside. How could I write a code that does the same in Java? I imagine I could return a class that encapsulates both values, but that seems really cumbersome.
-
Bombe over 15 yearsThat will still not change the objects outside of the method, as Integers are immutable.
-
Alan Moore over 15 yearsWhere did you get the idea that Integer had an add() method? Anyway, as Bombe pointed out, Integers are immutable, which leaves you with the exact same problem (but with more typing).
-
Alan Moore over 15 yearsLooks like I should have addressed that comment to jinguy; he's the one who added those methods.
-
John Gardner over 15 yearsyou could pass in AtomicInteger instead, that's mutable. That isn't its intended purpose, but would almost make this method work.
-
Johannes Schaub - litb over 15 yearsthis won't work as object references are passed by value, as everything in java. read this. stackoverflow.com/questions/373419/…
-
Johannes Schaub - litb over 15 yearsit has nothing todo with whether or not Integer is immutable tho. .. ugg it was jinguy who added those code in test. well ok it had something todo with immutability before jinguy changed that answer :p now it solely has todo with pass-by-value instead of pass-by-reference :)