How do I write a date in app.config file?
Solution 1
Store the value in the config file:
<appSettings>
<add key="DateKey" value="2012-06-21" />
</appSettings>
Then to retrieve the value you van use:
var value = ConfigurationSettings.AppSettings["DateKey"];
var appDate = DateTime.Parse(value);
Solution 2
It's easy to use the Settings in Visual Studio. Simply add settings by opening the properties for your project then go to Settings
and click "The project does not contain a default settings file. Click here to create one.".
Go to the Settings and add a DateTime and define your date
.
To access the Setting in code you simply do this.
DateTime myDate = Properties.Settings.Default.MyDate;
Solution 3
Not sure If I fully understand your question. I think that you want to:
You can define date in your app.config in the appSettings section:
<appSettings>
<add key="DateX" value="21/06/2012"/>
</appSettings>
And retrieve AppSettings entry by doing something similar to this:
Datetime dateX;
System.Configuration.Configuration rootWebConfig1 = System.Web.Configuration.WebConfigurationManager.OpenWebConfiguration(null);
if (rootWebConfig1.AppSettings.Settings.Count > 0)
{
System.Configuration.KeyValueConfigurationElement customSetting = rootWebConfig1.AppSettings.Settings["DateX"];
if (customSetting != null)
{
dateX = Datetime.Parse(customSetting.Value);
}
}
You can check this MSDN link for more help.
Solution 4
Assuming you're referring to the <appSettings>
element then you're short-of-luck initially: each key is associated with a string value.
Therefore you can see you just need to serialize the DateTime value to a string, and then parse it back when you read it back.
If you don't care about your app.config being edited by humans in notepad, then I'd store the 64-bit ticks value as a string integer:
ConfigurationManager.AppSettings["date"] = myDateTime.Ticks.ToString(CultureInfo.InvariantCulture);
Read it back by doing this:
Int64 ticks = Int64.Parse( ConfigurationManager.AppSettings["date"], NumberStyles.Integer, CultureInfo.InvariantCulture );
DateTime myDateTime = new DateTime( ticks );
However if you do want to make it human-readable then use the roundtrip option:
// Serialise
ConfigurationManager.AppSettings["date"] = myDateTime.ToString("o"); // "o" is "roundtrip"
// Deserialise
DateTime myDateTime = DateTime.Parse( ConfigurationManager.AppSettings["date"], NumberStyles.Integer, CultureInfo.InvariantCulture ) );
A few notes:
- My code is advisory and rough. In reality you'd ensure all DateTime instances are in UTC first and then apply an timezone offsets if necessary.
- You'd check if AppSettings contains a key named "date" first, and if not return a default or zero-equivalent answer.
- You'd also avoid .Parse methods and use TryParse instead and handle error conditions as appropriate for your application.
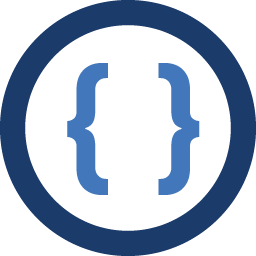
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I need to be able to define a date in app.config file. How do I do this and then retrieve it using c#?