How do routes in FOSRestBundle work?
Please follow the next URL to read the official documentation: http://symfony.com/doc/master/bundles/FOSRestBundle/index.html
To start with this bundle, I would suggest following the single restful controller documentation: http://symfony.com/doc/master/bundles/FOSRestBundle/5-automatic-route-generation_single-restful-controller.html
You will also find clear examples (https://github.com/liip/LiipHelloBundle) about what this bundle can offer.
Few things from the snippets you have posted drew my attention:
The visibility of your controller method is protected whereas it should be public (http://symfony.com/doc/current/book/controller.html)
public function postDatasetAction(Request $request) {
// your code
}
The "routing.yml" file created to configure your route shall contain the name of the aforementioned controller method (postDatasetAction instead of DatasetAction):
# routing.yml
data_query:
type: rest
pattern: /dataset
defaults: {_controller: DataAPIBundle:Dataset:postDatasetAction, _format: json }
requirements:
_method: POST
Please find below an example to setup a route like :
get_items GET ANY ANY /items.{json}
# config.yml
fos_rest:
allowed_methods_listener: true
format_listener:
default_priorities: ['json', html, '*/*']
fallback_format: json
prefer_extension: true
param_fetcher_listener: true
routing_loader:
default_format: json
view:
formats:
json: true
mime_types:
json: ['application/json', 'application/x-json']
force_redirects:
html: true
view_response_listener: force
# routing.yml
categories:
type: rest
resource: Acme\DemoBundle\Controller\ItemController
<?php
namespace Acme\DemoBundle\Controller
use FOS\RestBundle\Request\ParamFetcher;
use FOS\RestBundle\Controller\Annotations as Rest;
class ItemController
{
/**
* Get items by constraints
*
* @Rest\QueryParam(name="id", array=true, requirements="\d+", default="-1", description="Identifier")
* @Rest\QueryParam(name="active", requirements="\d?", default="1", description="Active items")
* @Rest\QueryParam(name="from", requirements="\d{4}-\d{2}-\d{2}", default="0000-00-00", description="From date")
* @Rest\QueryParam(name="to", requirements="\d{4}-\d{2}-\d{2}", default="0000-00-00", description="End date")
* @Rest\QueryParam(name="labels", array=true, requirements="\d+", default="-1", description="Labels under which items have been classifed")
*
* @Rest\View()
*
* @param ParamFetcher $paramFetcher
*/
public function getItemsAction(ParamFetcher $paramFetcher) {
$parameters = $paramFetcher->all();
// returns array which will be converted to json contents by FOSRestBundle
return $this->getResource($parameters);
}
}
P.S. : You will need to add a view to display the resource as an HTML page
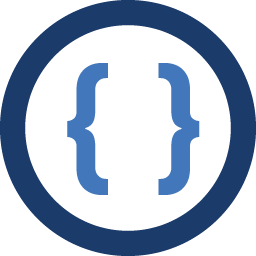
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
Can someone clearly explain how routes are supposed to be configured for REST requests using FOSRest? Every tutorial seems to do it differently.
My Controller:
<?php namespace Data\APIBundle\Controller; use Symfony\Bundle\FrameworkBundle\Controller\Controller; use Symfony\Component\HttpFoundation\Request; class DatasetController extends Controller{ protected function postDatasetAction(Request $request){ //Query here }
The URL should look something like this: Symfony/web/app_dev.php/api/dataset. So I thought the routes should be something like...
app/config/routes.yml
data_api: resource: "@DataAPIBundle/Resources/config/routing.yml" prefix: /api type: rest
And....
Data/APIBundle/Resources/config/routing.yml
data_query: type: rest pattern: /dataset defaults: {_controller: DataAPIBundle:Dataset:datasetAction, _format: json } requirements: _method: POST
-
Jon L. about 11 yearsThe documentation and Liip bundle are both fairly limited in terms of actual content/examples. Post some examples of advanced usage of this bundle, I'll award you the bounty. For instance, custom route usage (Route, Get, Post, etc), the "magic" addition of the request format to routes, a solid example of how to use the view listener for json & html while returning an array/object from your action.
-
Kioko Kiaza about 11 yearsthe // "post_dataset" [POST] /dataset part
-
Felix Aballi almost 10 yearsI think is great the solution, but I'm looking for others where params injection occurs, similar to .yml files (%the_date%) but in FOS Controller's action annotation instead, that param will be self initialized. Could be done? Please, check the full question: github.com/FriendsOfSymfony/FOSRestBundle/issues/774
-
Thierry M.S. almost 10 years@Félix, you might want to overload the
QueryParam
class. -
Felix Aballi almost 10 years@ThierryMarianne, where can i find more facts?
-
Thierry M.S. almost 10 years@Félix, you might want to study the original
QueryParam
github.com/FriendsOfSymfony/FOSRestBundle/blob/master/… -
Felix Aballi almost 10 years@ThierryMarianne, I read it. But it doesn't tell how to extend Param class. Any example available?
-
Thierry M.S. almost 10 years@Félix Tests of ParamFetcher and other classes can also be of great help to understand how the classes relate altogether and how they could be extended github.com/FriendsOfSymfony/FOSRestBundle/blob/master/Tests/…
-
Tom T over 9 yearsI think that this was just added to the documentation to better illustrate the generated routes by the package. if this is wrong please correct me.
-
Pedro Luz about 9 yearsAll those links are completely outdated and are irrelevant
-
Joe over 7 yearsThat's simply a comment not annotations.