How do you import a javascript package from a cdn/script tag in React?
Solution 1
Go to the index.html file and import the script
<script src="https://cdn.dwolla.com/1/dwolla.js"></script>
Then, in the file where dwolla is being imported, set it to a variable
const dwolla = window.dwolla;
Solution 2
This question is getting older, but I found a nice way to approach this using the react-helmet
library which I feel is more idiomatic to the way React works. I used it today to solve a problem similar to your Dwolla question:
import React from "react";
import Helmet from "react-helmet";
export class ExampleComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
myExternalLib: null
};
this.handleScriptInject = this.handleScriptInject.bind(this);
}
handleScriptInject({ scriptTags }) {
if (scriptTags) {
const scriptTag = scriptTags[0];
scriptTag.onload = () => {
// I don't really like referencing window.
console.log(`myExternalLib loaded!`, window.myExternalLib);
this.setState({
myExternalLib: window.myExternalLib
});
};
}
}
render() {
return (<div>
{/* Load the myExternalLib.js library. */}
<Helmet
script={[{ src: "https://someexternaldomain.com/myExternalLib.js" }]}
// Helmet doesn't support `onload` in script objects so we have to hack in our own
onChangeClientState={(newState, addedTags) => this.handleScriptInject(addedTags)}
/>
<div>
{this.state.myExternalLib !== null
? "We can display any UI/whatever depending on myExternalLib without worrying about null references and race conditions."
: "myExternalLib is loading..."}
</div>
</div>);
}
}
The use of this.state
means that React will automatically be watching the value of myExternalLib and update the DOM appropriately.
Credit: https://github.com/nfl/react-helmet/issues/146#issuecomment-271552211
Solution 3
for typescript developers
const newWindowObject = window as any;
// cast it with any type
let pushNotification = newWindowObject.OneSignal;
// now OneSignal object will be accessible in typescript without error
Solution 4
You can't require or import modules from a URL.
What you can do is make an HTTP request to get the script content & execute it, as in the answer for how to require from URL in Node.js
But this would be a bad solution since your code compilation would depend on an external HTTP call.
A good solution would be to download the file into your codebase and import it from there. You could commit the file to git if the file doesn't change much & are allowed to do it. Otherwise, a build step could download the file.
Related videos on Youtube
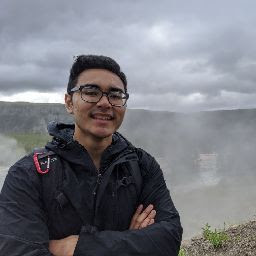
Jeremy Herzog
Updated on July 08, 2022Comments
-
Jeremy Herzog almost 2 years
I'd like to import this javascript package in React
<script src="https://cdn.dwolla.com/1/dwolla.js"></script>
However, there is no NPM package, so I can't import it as such:
import dwolla from 'dwolla'
or
import dwolla from 'https://cdn.dwolla.com/1/dwolla.js'
so whenver I try
dwolla.configure(...)
I get an error saying that dwolla is undefined. How do I solve this?
Thanks
-
Saad almost 7 yearsIt looks like there is an official
dwolla
npm module. You can read about it here. You can install it by doingnpm install dwolla-v2
. -
Jeremy Herzog almost 7 yearsThat's not the front end module for account verification though
-
Saad almost 7 yearsAh okay, my mistake.
-
-
technazi over 4 yearsWhat if the file is firebase-app.js?
-
Adam about 3 years@technazi try
window["firebase-app"]
. Or just do aconsole.log(window)
and search for your library. -
KevynTD about 2 yearsJust updating, today its possible: stackoverflow.com/a/34607405/6227108