How does this comparator work?
Solution 1
What is a Comparable interface? It is an interface containing single method:
compareTo(T o)
providing capability for Java to comprare your object with any other. Basically you would like to compare your object with those of the same kind (class). So there is a usual check in compareTo implementation:
if (o instanceof vehicles) {
Vehicles v = (Vehicles)o;
// compare
} else {
return false;
}
Then, in compare section you should decide, based on your business-rules, whether other vehicle is equals, more of less to your object.
- 0 - if they are equal;
- 1 - if your object is greater;
- -1 - if your object is lesser.
All that simple!
Solution 2
It uses bubble sort, sometimes referred to as sinking sort, is a simple sorting algorithm that repeatedly steps through the list to be sorted, compares each pair of adjacent items and swaps them if they are in the wrong order. The pass through the list is repeated until no swaps are needed, which indicates that the list is sorted.
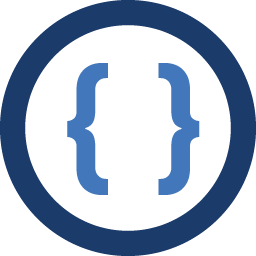
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
package vehicles_order; import java.util.ArrayList; import java.util.Collections; import java.util.Iterator; public class vehicles implements Comparable { String Vehicle; String Make; String Type; double Cost; public static void main(String[] args){ ArrayList cars = new ArrayList(); cars.add(new vehicles ("CAR","Peugot","3008",12500.00)); cars.add(new vehicles ("CAR","BMW","316",4995.00)); cars.add(new vehicles ("CAR","Ford","Fiesta",2995.00)); Collections.sort(cars); Iterator itr = cars.iterator(); while(itr.hasNext()){ Object element = itr.next(); System.out.println(element + "\n"); } } public vehicles(String vehicle, String make, String type, double cost){ Vehicle = vehicle; Make = make; Type = type; Cost = cost; } public String toString() { return "Vehicle: " + Vehicle + "\n" + "Make: " + Make + "\n" + "Type: " + Type + "\n" + "Cost: " + Cost; } public int compareTo(Object o1) { if (this.Cost == ((vehicles) o1).Cost) return 0; else if ((this.Cost) > ((vehicles) o1).Cost) return 1; else return -1; } }
My comparator is at the bottom and I just wondering how it actually works. Im guessing its like a stack, where when it returns 1 it moves up the stack if its -1 moves down.
Also, can anyone tell me how id go about ordering the cars via a different method. For example, store the cost in a temp highest value and check if the new value is higher than the current one