How exactly does queryForMap() and queryForList() methods provided by the Spring JdbcTemplate work internally?
- You'll get an exception. You could simply write a test to confirm it.
- The documentation of the method says: The query is expected to be a single row query, so yes, the query is supposed to return a single row. It also says: Returns: the result Map (one entry for each column, using the column name as the key), which should clear your doubts and refute your wrong assumption.
- The documentation says: The results will be mapped to a List (one entry for each row) of Maps (one entry for each column using the column name as the key), which, once again should be enough to clear your doubts and refute your wrong assumption.
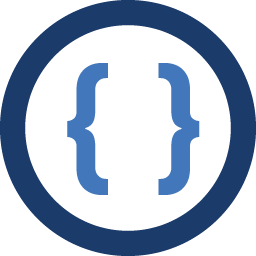
Admin
Updated on August 03, 2022Comments
-
Admin over 1 year
I am studying for the Spring Core certification and I have some doubt related to the use of the JdbcTemplate use.
I know that JdbcTemplate gives me different methods to perform queries (that are written in SQL).
So I have the following methods:
1) queryForObject():
public long getPersonCount() { String sql = “select count(*) from PERSON”; return jdbcTemplate.queryForObject(sql, Long.class); }
So in this method I specify 2 parameters that represent respectively the SQL statement and the class type of the returned object. In the previous case it is specified that the result of the query is returned into a Long object.
What happens if the result returned from the query doesn't match with the specified type? (for example if the query returns a String and I have specified a Long as parameter of the queryForObject() method? How are situations like this handled?
2) queryForMap():
public Map getPersonInfo(int id) { String sql = “select * from PERSON where id=?”; return jdbcTemplate.queryForMap(sql, id); }
Reading the documentation it seems to me that this is used only when it is known that my query returns a single row. Is it correct?
So now I have a doubt related the use of the queryForMap() method.
I know that the Map interface stores objects using system.
So a Map is thought to store multiple couples. So I think that this could store multiple rows: the key of the Map is the key of a row of my table and the value contains the values of the other columns (into an object).
But it seems that the logic of the queryForMap() method is pretty different. How does it work exactly?
Maybe it returns a Map that works in this way:
key: contains the key of the primary key of the table and with this key are associated multiple values related to the content of the other fields of my table. Or what?
3) queryForList():
public List getAllPersonInfo() { String sql = “select * from PERSON”; return jdbcTemplate.queryForList(sql); }
Reading the documentation it seems to me that it is used when I expect that my query returns multiple rows. And it seems to me that the method output is a List of Map (so according to the previous method each Map represents a single returned row** (but the previous doubt about how a row is stored into a Map persists).
Can you help me to understand deeply how these methods exactly work?
Tnx