How I can work with Amazon's Dynamodb Local in Node?
22,117
Solution 1
You should follow this blog post to setup your DynamoDB Local, an then you can simply use this code:
var AWS= require('aws-sdk'),
dyn= new AWS.DynamoDB({ endpoint: new AWS.Endpoint('http://localhost:8000') });
dyn.listTables(function (err, data)
{
console.log('listTables',err,data);
});
Solution 2
For Node please do as below:
const AWS = require('aws-sdk');
const AWSaccessKeyId = 'not-important';
const AWSsecretAccessKey = 'not-important';
const AWSregion = 'local';
const AWSendpoint = 'http://localhost:8000' // This is required
AWS.config.update({
accessKeyId: AWSaccessKeyId,
secretAccessKey: AWSsecretAccessKey,
region: AWSregion,
endpoint: AWSendpoint
});
Ensure that DynamodDB is running on port 8000.
Solution 3
Here is how I do it, same code works local or inside AWS.
Simply leverage existence of env var DYNAMO_LOCAL_ENDPT="http://localhost:8000"
import { DynamoDB, Endpoint } from 'aws-sdk';
const ddb = new DynamoDB({ apiVersion: '2012-08-10' });
if (process.env['DYNAMO_LOCAL_ENDPT']) {
ddb.endpoint = new Endpoint(process.env['DYNAMO_LOCAL_ENDPT']);
}
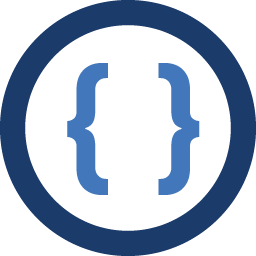
Author by
Admin
Updated on January 31, 2022Comments
-
Admin over 2 years
Amazon offers a local simulator for their Dynamodb product but the examples are only in PHP.
These examples mention passing the parameter "base_url" to specify that you're using a local Dynamodb, but that returns this error in Node:
{ [UnrecognizedClientException: The security token included in the request is invalid.] message: 'The security token included in the request is invalid.', code: 'UnrecognizedClientException', name: 'UnrecognizedClientException', statusCode: 400, retryable: false }
How do I get Dynamodb_local working in Node?