How merge two objects array in angularjs?
Solution 1
You can use angular.extend(dest, src1, src2,...);
In your case it would be :
angular.extend($scope.actions.data, data);
See documentation here :
https://docs.angularjs.org/api/ng/function/angular.extend
Otherwise, if you only get new values from the server, you can do the following
for (var i=0; i<data.length; i++){
$scope.actions.data.push(data[i]);
}
Solution 2
This works for me :
$scope.array1 = $scope.array1.concat(array2)
In your case it would be :
$scope.actions.data = $scope.actions.data.concat(data)
Solution 3
$scope.actions.data.concat is not a function
same problem with me but i solve the problem by
$scope.actions.data = [].concat($scope.actions.data , data)
Solution 4
Simple
var a=[{a:4}], b=[{b:5}]
angular.merge(a,b) // [{a:4, b:5}]
Tested on angular 1.4.1
Related videos on Youtube
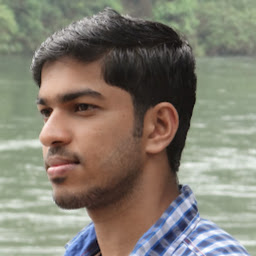
Muhammed Shihab
Updated on September 14, 2020Comments
-
Muhammed Shihab over 3 years
I want to append following object array with existing one in angulajs for implementing load more feature.
ie,appending AJAX response with existing one each time.
I have one variable,
$scope.actions
which contains followingJSON
data,{ "total": 13, "per_page": 2, "current_page": 1, "last_page": 7, "next_page_url": "http://invoice.local/activities/?page=2", "prev_page_url": null, "from": 1, "to": 2, "data": [ { "id": 2108, "action_type_id": 202, "user_id": 1 }, { "id": 2108, "action_type_id": 202, "user_id": 1 } ] }
I want to append following
JSON
response each time this variable.{ "data": [ { "id": 2108, "action_type_id": 202, "user_id": 1 }, { "id": 2108, "action_type_id": 202, "user_id": 1 } ] }
I have tried with
$scope.actions.data.concat(data.data);
but it is not working and getting following error message
$scope.actions.data.concat is not a function
-
adeneo almost 9 yearsAngular has both
extend
andcopy
that copies objects -
Sagar Naliyapara almost 9 years
-
charlietfl almost 9 yearsSince new variable is exactly the same as
data
property in first sample, some clarification would help -
adeneo almost 9 years
-
-
Deblaton Jean-Philippe almost 9 yearscan't you give more info?
-
Muhammed Shihab almost 9 years`angular.extend($scope.actions, data); it is replacing not appending :(
-
Muhammed Shihab almost 9 yearsWhen trying second method,getting error
Error: $scope.actions.data.push is not a function
-
MuntingInsekto over 8 yearsI think you should try to do this
angular.extend({}, $scope.actions.data, data);
. docs.angularjs.org/api/ng/function/angular.extend. This helps me a lot and this also works for me. -
DShah over 8 yearsangular.extend and angular.merge is just replacing the values and not merging. Any idea why it happens?
-
Mark about 8 yearsangular.extend() is a shallow copy and will fail in the general case (arrays of arbitrarily complex objects). angular.merge() implements a deep copy, but also failed during cursory testing... I opted for the direct solution: src.forEach(function (x) { dst.push(x); }); to merge src into dst.
-
serge over 7 yearsUnlike extend(), merge() recursively descends into object properties of source objects, performing a deep copy.
-
benjaminhull almost 5 years@davidH This is only the correct answer if you want to just plonk two arrays together. Ideal if the keys of the array are just an arbitrary index number. However, for the actual example in the question, each item is being returned with a specific ID, so merge or extend are better here to avoid duplicates in the resulting array.
-
rahim.nagori almost 5 yearsThis is not working for me. I'm using $scope.actions.data in an ng-repeat, the value in console is updating but not in the view file.