How should I create a child window in win32 while programming with C++?
Solution 1
Roughly speaking, in the handler for the parent, where you wish to create the child, you call CreateWindow
, passing in the window for the parent as the hwndParent
- probably, you also want to set certain styles on the child such as WS_CHILD
. Your interaction with the child window then depends on the type of the window you created. Some windows (such as buttons) are designed to work as child windows, so they send a lot of notification messages, so you would set up your parent to listen for those notification messages.
Solution 2
I'd highly recommend having a read through Charles Petzold's "Programming Windows" if you can obtain a copy.
Otherwise, to answer your question, pass the parent window's handle as the parent when you create the child window (using either CreateWindow or CreateWindowEx):
HWND CreateWindowEx
(
DWORD dwExStyle,
LPCTSTR lpClassName,
LPCTSTR lpWindowName,
DWORD dwStyle,
int x,
int y,
int nWidth,
int nHeight,
HWND hWndParent, /// pass the parent window handle here
HMENU hMenu,
HINSTANCE hInstance,
LPVOID lpParam
);
..As 1800 Info has also stated, perhaps also set the WS_CHILD style (more oon Window Styles here). This is just the rudimentary plumbing, really..
Can you be a bit more specific when you say "control the child window..."?
Solution 3
It Is 2018 by now.. doing some retro work on this I found SetParent() to come out handy, to assure a child window remains inside the client region of the parent.. Before SetParent() the child need not be registered as a child. In CreateWindowEx the parent handle can be NULL at first. Style WS_CHILD is not needed, but WS_CLIPSIBLINGS comes out handy, it avoids flickering.
This is my code for creating a child window:
HWND hwnd = CreateWindowExA(0, "WindowOfDLL", ctitle, WS_SIZEBOX | WS_CLIPSIBLINGS, CW_USEDEFAULT, CW_USEDEFAULT, 400, 300, NULL, hMenu, inj_hModule, NULL);
SetParent(hwnd, hwndparent);
ShowWindow(hwnd, SW_SHOWNORMAL);
I've seen no problems (yet) with threading in Win10. Each of below child windows is created in a DLL which manages the assembly. When a child window is created, the DLL adds a new member to the assembly and launches a thread, which will serve the child window and performs above code in its initialisation.
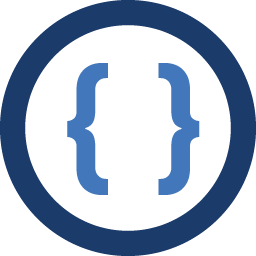
Admin
Updated on January 19, 2020Comments
-
Admin over 4 years
i'm new to C++ as well as for windows programming..
i have created a window using msdn
CreateWindow()
functionwhich works correctly..now i would like to create a child window...the parent window should control the child window...
Any helps sample code regarding this .
Thanks in advance
-
Brad B. over 5 yearsSo I'm thinking about posting my own question related to getting a child window to work because I have not been able to get one to load. I've used your line with some changes - HWND hwnd = CreateWindowExA(0, "WindowOfDLL", "child", WS_SIZEBOX | WS_CLIPSIBLINGS, CW_USEDEFAULT, CW_USEDEFAULT, 400, 300, NULL, NULL, hInstance, NULL); - gave explicit child name, removed the menu, and pointed at my hInstance. I have been unsuccessful in getting one to load. Could you post more of your code so I can see what I'm doing wrong?