How to access and pass parameters to the modules of an Android Instant App
Solution 1
Instant Apps and Deep Linking
Instant Apps rely on App Links to work, and App Links are just one type of deep link. So deep linking is still possible for Instant Apps, and is in fact absolutely critical to how they function. However, URI scheme deep linking (which is still very prevalent in Android apps) is not supported.
The difference between a regular app and an Instant App is that the device will only load a single Activity in response to the App Link the user clicks, instead of needing to download the full package through the Play Store. It's a more seamless experience for the user, but the underlying technology works the same way.
Passing Custom Parameters
If the user clicks an App Links-enabled URL like http://www.myinstantappexample.com/onlyviewmap/?x=0.000&y=0.000
, you will get that entire string back inside the app after it opens. You'll have to parse out the x
and y
variables yourself, but they will be available to you. Something like this:
Uri data = this.getIntent().getData();
if (data != null && data.isHierarchical()) {
String uri = this.getIntent().getDataString();
Log.i("MyApp", "Deep link clicked " + uri);
}
You'll just need to manipulate the uri
value to find what you need.
Alternative Approach to Custom Parameters
Alternatively, you can use Branch.io (full disclosure: I'm on the Branch team) to power your links. We have full support for Instant Apps, and this allows you to work with a much more friendly data format. We let you create links like this, to control every part of the behavior:
branch.link({
tags: [ 'tag1', 'tag2' ],
channel: 'facebook',
feature: 'dashboard',
stage: 'new user',
data: {
x: '0.000',
y: '0.000',
'$desktop_url': 'http://myappwebsite.com',
'$ios_url': 'http://myappwebsite.com/ios',
'$ipad_url': 'http://myappwebsite.com/ipad',
'$android_url': 'http://myappwebsite.com/android',
'$og_app_id': '12345',
'$og_title': 'My App',
'$og_description': 'My app\'s description.',
'$og_image_url': 'http://myappwebsite.com/image.png'
}
}, function(err, link) {
console.log(err, link);
});
In return you get a URL like http://myappname.app.link/iDdkwZR5hx
, and then inside the app after the link is clicked, you'll get something that looks like this:
{
tags: [ 'tag1', 'tag2' ],
channel: 'facebook',
feature: 'dashboard',
stage: 'new user',
data: {
x: '0.000',
y: '0.000'
}
}
Solution 2
If I want it to point to a specific set of coordinates how would the link be composed?
It's up to you how to include any additional info in the URL. It could be via URL parameters or in the path itself. Eg.
https://www.myinstantappexample.com/location/2/user/5
https://www.myinstantappexample.com/onlyviewmap/?x=1.2&y=3.4
You then parse the URL in the receiving Activity
. The Uri
class includes a number of helper methods such as getQueryParameter()
and getPathSegments()
to make this easier.
For example, to parse this URL:
https://www.myinstantappexample.com/onlyviewmap/?x=1.2&y=3.4
You would do something like this in your Activity
:
Uri uri = getIntent().getData();
String x;
String y;
if (uri != null) {
x = uri.getQueryParameter("x"); // x = "1.2"
y = uri.getQueryParameter("y"); // y = "3.4"
}
if (x != null && y != null) {
// do something interesting with x and y
}
Solution 3
In order to do that, you have to use the "app links assistant" in Tools->App Links Assistant
Then check your links and, in the Path selector, check that the "pathPrefix" option is selected.
Then at the bottom of the OnCreate method of your activity (which is related to the link you recently edited) add this code:
Intent appLinkIntent = getIntent();
String appLinkAction = appLinkIntent.getAction();
Uri appLinkData = appLinkIntent.getData();
// then use appLinkData.getQueryParameter("YourParameter")
You can test and debug this, using the "editConfigurations" option, just open that window and edit your instantApp module (the one launched with the Link you recently edited) and in the URL field add the URL parameters that you need. (then just run that module :D )
Hope this to be helpful.
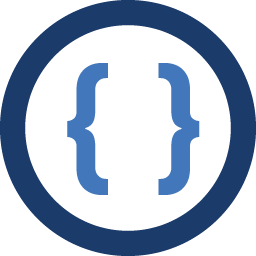
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
With normal installed apps it's possible to use the technique of Deep Linking in order to not only open a specific application from an URL but also to redirect it to a specific section/function such as a specific Facebook post or specific coordinates on a map.
Since I've read that with Instant Apps this won't be possible because links already point to the specific module to download and run, how would it be possible to access not only the said module but also pass it some parameters?
For example:
This is the link from which the view-only module of my map application will be downloaded: "www.myinstantappexample.com/onlyviewmap"
If I want it to point to a specific set of coordinates how would the link be composed? Will it be like this: "www.myinstantappexample.com/onlyviewmap/?x=0.000&y=0.000" ?
From what I've been able to find google doesn't cover this aspect and I really can't wrap my head around it.
-
Dan Chaltiel over 6 yearsHard choice... first syntax is more beautiful but you cannot call arguments by name with
getPathSegments()
-
Vadim Kotov about 5 yearsIf you're going to test you deeplinks with multiple parameters via adb, refer to this - stackoverflow.com/questions/35645414/…
-
Jonas Simonaitis over 2 yearsWhat about the case, when the user has never enabled instant experience on Google Play Store app and you still need to pass some data via URL
https://play.google.com/store/apps/details?id=<package_name>&launch=true
? How would you get the data with google play link? -
Michel Gokan Khan over 2 years@AlexBauer I tried your "Branch Monster Factoy" demo, but the link to a monster only works if the app is installed. Is there any way that I can use that link to open the app with the shared monster using the Instant version of the app (without need for installing the app)?