How to access Parent class function/control from a child user control loaded in a pannel
Solution 1
This may helps:
public partial class Form1 : Form
{
//Other codes
private void loadlogin()
{
login log = new login(this); //CHANGE HERE
mainPannel.Controls.Clear();
mainPannel.Controls.Add(log);
}
//Other codes
}
And
public partial class login : UserControl
{
Form1 _parent; //ADD THIS
public login(Form1 parent)
{
InitializeComponent();
this._parent = parent; //ADD THIS
}
public void button1_Click_1(object sender, EventArgs e)
{
this._parent.mytest(); //CALL WHAT YOU WANT
}
}
Solution 2
Ok, the above answer will work but it is not good coding practice to pass in a "Parent Form" to a user control. UserControls should be located in a separate project from your forms project and your forms project should reference your control project in order to gain visiblity to them. Lets say for instance that you want this UserControl to be nested in another UserControl at some point. Your code no longer works without overloading the constructor. The better solution would be to use an event. I have provided an implementation using a CustomEventArg. By doing this, your login UserControl can sit on anything and still work. If the functionality to change the parents text is not requried, simply do not register with the event. Here is the code, hope this helps someone.
Here is the form code:
public Form1()
{
InitializeComponent();
loadlogin();
}
private void loadlogin()
{
login log = new login();
//Registers the UserControl event
log.changeParentTextWithCustomEvent += new EventHandler<TextChangedEventArgs>(log_changeParentTextWithCustomEvent);
mainPannel.Controls.Clear();
mainPannel.Controls.Add(log);
}
private void log_changeParentTextWithCustomEvent(object sender, TextChangedEventArgs e)
{
this.Text = e.Text;
}
Here is the "login" UserControl code (in my test solution, just a userControl with a button)
public partial class login : UserControl
{
//Declare Event with CustomArgs
public event EventHandler<TextChangedEventArgs> changeParentTextWithCustomEvent;
private String customEventText = "Custom Events FTW!!!";
public login()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
//Check to see if the event is registered...
//If not then do not fire event
if (changeParentTextWithCustomEvent != null)
{
changeParentTextWithCustomEvent(sender, new TextChangedEventArgs(customEventText));
}
}
}
And finally, the CustomEventArgs class:
public class TextChangedEventArgs : EventArgs
{
private String text;
//Did not implement a "Set" so that the only way to give it the Text value is in the constructor
public String Text
{
get { return text; }
}
public TextChangedEventArgs(String theText)
: base()
{
text = theText;
}
}
Writing your controls in this fashion, with no visibility to Forms will allow your UserControls to be completely reusable and not bound to any type or kind of parent. Simply define the behaviors a UserControl can trigger and register them when necessary. I know this is an old post but hopefully this will help someone write better UserControls.
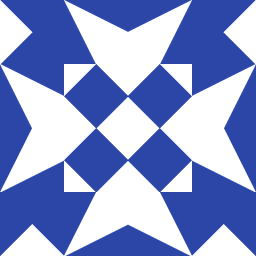
Surja
Updated on June 26, 2022Comments
-
Surja 12 months
I have Main Form which contains a Panel which loads different Usercontrol into the panel. Now i need to access functions in the Main Form from the UserControl. Below i have given my code;
This is my main Windows form class:
public partial class Form1 : Form { public Form1() { InitializeComponent(); loadlogin(); } private void loadlogin() { login log = new login(); mainPannel.Controls.Clear(); mainPannel.Controls.Add(log); } public void mytest() { } }
As you can see i am loading a usercontrol to the mainPannel. Now lets look at the usercontrol:
public partial class login : UserControl { string MyConString = ""; public login() { InitializeComponent(); } public void button1_Click_1(object sender, EventArgs e) { //I want to call the parent function mytest(); HOW???? } }
I want to access mytest() function when button1 us clicked. I have tried alot of other solution but i am still confused. I have used:
Form my = this.FindForm(); my.Text = "asdasfasf";
This gives me reference to parent and i can change the form text but how can i access its functions???