How to add a Hint in spinner
14,413
There is not any default way to display hint in spinner. For this you need to add one item manually in the array like below.
val myStrings = arrayOf("Select","One", "Two" , "Three", "Four")
Now, Define custom Adapter for the Spinner and disable the first item like below.
@Override
public boolean isEnabled(int position) {
if (position == 0) {
// Disable the first item from Spinner
// First item will be use for hint
return false;
} else {
return true;
}
}
You can change the color like below
@Override
public View getDropDownView(int position, View convertView,
ViewGroup parent) {
View view = super.getDropDownView(position, convertView, parent);
TextView tv = (TextView) view;
if (position == 0) {
// Set the hint text color gray
tv.setTextColor(Color.GRAY);
} else {
tv.setTextColor(Color.BLACK);
}
return view;
}
For more info please visit:-
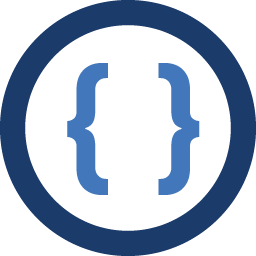
Author by
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
XML code of spinner:
<Spinner android:id="@+id/mySpinner" https://stackoverflow.com/questions style="@style/Widget.AppCompat.DropDownItem.Spinner" android:layout_width="match_parent" android:layout_height="70dp" />`
.kotlin:
val myStrings = arrayOf("One", "Two" , "Three", "Four") mySpinner.adapter = ArrayAdapter(this, android.R.layout.simple_spinner_dropdown_item, myStrings) mySpinner.onItemSelectedListener = object : AdapterView.OnItemSelectedListener { override fun onNothingSelected(parent: AdapterView<*>?) { TODO("not implemented") //To change body of created functions use File | Settings | File Templates. } override fun onItemSelected(parent: AdapterView<*>?, view: View?, position: Int, id: Long) { TODO("not implemented") //To change body of created functions use File | Settings | File Templates. } }}
Equal to the "hint" option in Edittext, I need a default text in a Spinner.