How to add an if else condition for login?
I had an issue like this, we solved it using this function:
Future<String> checkBothUserBasesForTheUser(String uid) async {
DocumentSnapshot _doc = await db.collection('users').doc(uid).get();
if (_doc.exists) return 'user';
DocumentSnapshot _userDoc =
await db.collection('agencyUsers').doc(uid).get();
if (_userDoc.exists || _doc.exists)
return 'agency';
else
return null;
}
*** you should know that you will have multiple reads each time someone logins.
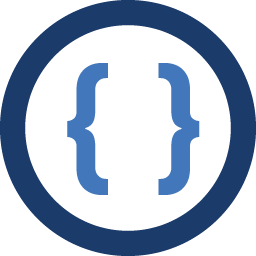
Admin
Updated on January 01, 2023Comments
-
Admin over 1 year
I am facing a problem in regards to logging in through Firebase in flutter app. In the app, I have 2 types of users who can log in : an agency and a normal user. (In cloud firestore, there are 2 collections named users and agencies with unique uid and aid respectively.)
The normal user can log in properly and get redirected to their homepage but it does not work for the agency. However, the agency can register an account and it is saved into the firebase server under agencies collection and it promptly gets redirected to its homepage named AgencyHomepage().
But for login, I was wondering if it would be possible to put a condition to recognize if the person trying to login is an agency or normal user, then redirect them to their respective homepages?
void signIn(String email, String password) async { if (_formKey.currentState!.validate()) { await _auth .signInWithEmailAndPassword(email: email, password: password) .then((uid) => { Fluttertoast.showToast( timeInSecForIosWeb: 2, gravity: ToastGravity.CENTER, msg: "Login Successful"), Navigator.of(context).pushReplacement( MaterialPageRoute(builder: (context) => MainPage())), }) .catchError((e) { Fluttertoast.showToast( timeInSecForIosWeb: 3, gravity: ToastGravity.CENTER, msg: "The email or password is invalid. Please check and try again.", ); }); } }
The code above is the function for signing in for users and it gets triggered when you click on the login button. For agency, I want to the MainPage() to be AgencyHomepage() instead.
Full Code:
class _LoginState extends State<Login> { GoogleAuth authentication = GoogleAuth(); final _formKey = GlobalKey<FormState>(); final TextEditingController emailController = new TextEditingController(); final TextEditingController passwordController = new TextEditingController(); final _auth = FirebaseAuth.instance; bool _showPwd = true; @override Widget build(BuildContext context) { final emailField = TextFormField( autofocus: false, controller: emailController, keyboardType: TextInputType.emailAddress, validator: (value) { if (value!.isEmpty) { return ("Please enter your email"); } // reg expression for email validation if (!RegExp("^[a-zA-Z0-9+_.-]+@[a-zA-Z0-9.-]+.[a-z]") .hasMatch(value)) { return ("Please enter a valid email"); } return null; }, onSaved: (value) { emailController.text = value!; }, textInputAction: TextInputAction.next, decoration: InputDecoration( prefixIcon: Icon(Icons.mail), contentPadding: EdgeInsets.fromLTRB(20, 15, 20, 15), hintText: "Email", border: OutlineInputBorder( borderRadius: BorderRadius.circular(10), ), )); final passwordField = TextFormField( autofocus: false, controller: passwordController, obscureText: _showPwd, validator: (value) { RegExp regex = new RegExp(r'^.{6,}$'); if (value!.isEmpty) { return ("Password is required"); } if (!regex.hasMatch(value)) { return ("Password needs to have minimum 6 characters"); } }, onSaved: (value) { passwordController.text = value!; }, textInputAction: TextInputAction.done, decoration: InputDecoration( prefixIcon: Icon(Icons.vpn_key), suffixIcon: IconButton( icon: Icon(_showPwd ? Icons.visibility : Icons.visibility_off), onPressed: () { setState(() { _showPwd = !_showPwd; }); }), contentPadding: EdgeInsets.fromLTRB(20, 15, 20, 15), hintText: "Password", border: OutlineInputBorder( borderRadius: BorderRadius.circular(10), ), )); final loginButton = Material( elevation: 5, borderRadius: BorderRadius.circular(30), color: Color(0xFF003893), child: MaterialButton( padding: EdgeInsets.fromLTRB(20, 15, 20, 15), minWidth: 300, onPressed: () { signIn(emailController.text, passwordController.text); }, child: Text( "Login", textAlign: TextAlign.center, style: TextStyle( fontSize: 16, color: Colors.white, fontWeight: FontWeight.bold), )), ); return Scaffold( backgroundColor: Colors.white, appBar: AppBar( centerTitle: true, title: RichText( text: TextSpan( style: Theme.of(context) .textTheme .headline6! .copyWith(fontWeight: FontWeight.bold), children: [ TextSpan( text: "Login", style: TextStyle(color: Colors.black), ), ], ), ), backgroundColor: Colors.transparent, elevation: 0, leading: IconButton( icon: Icon(Icons.arrow_back, color: Colors.black), onPressed: () { Navigator.of(context).pop(); }, ), ), body: SingleChildScrollView( child: Container( color: Colors.white, child: Padding( padding: const EdgeInsets.all(36.0), child: Form( key: _formKey, child: Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: <Widget>[ SizedBox( height: 100, child: Image.asset( "assets/icons/temp.png", fit: BoxFit.contain, )), SizedBox(height: 40), emailField, SizedBox(height: 25), passwordField, SizedBox(height: 40), loginButton, SizedBox(height: 30), Row( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text("Don't have an account? "), GestureDetector( onTap: () { Navigator.pop(context); Navigator.push( context, MaterialPageRoute( builder: (context) => UserRegistration())); }, child: Text( "Sign Up", style: TextStyle( color: Colors.blueAccent, fontWeight: FontWeight.bold, fontSize: 15), ), ) ]), Padding( padding: const EdgeInsets.only(top: 30), child: TextButton.icon( style: TextButton.styleFrom( primary: Color(0xfF003893), ), onPressed: () async { await authentication.googleLogin(); }, label: Text('Sign In with Google'), icon: Image.asset('assets/icons/google_logo.png', height: 30, width: 30), ), ), ], ), ), ), ), ), ); } void signIn(String email, String password) async { if (_formKey.currentState!.validate()) { await _auth .signInWithEmailAndPassword(email: email, password: password) .then((uid) => { Fluttertoast.showToast( timeInSecForIosWeb: 2, gravity: ToastGravity.CENTER, msg: "Login Successful"), Navigator.of(context).pushReplacement( MaterialPageRoute(builder: (context) => MainPage())), }) .catchError((e) { Fluttertoast.showToast( timeInSecForIosWeb: 3, gravity: ToastGravity.CENTER, msg: "The email or password is invalid. Please check and try again.", ); }); } } }
-
Frank van Puffelen over 2 yearsSounds feasible. You can read try to read their document from the
agencies
collection and base your redirect on whether the document exists there. As a next step I'd recommend having a single collection for this (e.g.user_types
), so that you can always determine the user type from reading a single document.
-
-
Admin over 2 yearsHi, I added the function. Do I now have to call the checkBothUserBasesForTheUser in void signIn? I'm still new to flutter so I am not sure where the checkBothUserBasesForTheUser function has to be added in the code.