How to add an item in a collection using Linq and C#
26,738
Solution 1
You don't. LINQ is for querying, not adding. You add a new item by writing:
subscription.Add(new Subscription { Type = "Foo", Type2 = "Bar", Period = 1 });
(Note that you can't specify the property Type
twice in the same object initializer.)
This isn't using LINQ at all - it's using object initializers and the simple List<T>.Add
method.
Solution 2
I would suggest using List.Add()
:
subscription.Add(new Subscriptioin(...))
LINQ Union()
overkill by wrapping a single item by a List<>
instance:
subscriptions.Union(new List<Subscription> { new Subscriptioin(...) };
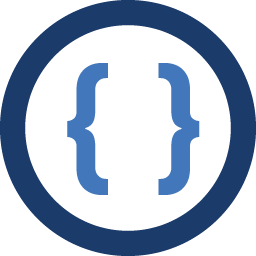
Author by
Admin
Updated on June 12, 2020Comments
-
Admin almost 4 years
I have a collection of objects. e.g.
List<Subscription> subscription = new List<Subscription> { new Subscription{ Type = "Trial", Type = "Offline", Period = 30 }, new Subscription{ Type = "Free", Type = "Offline", Period = 90 }, new Subscription{ Type = "Paid", Type = "Online", Period = 365 } };
Now I want to add one more item in this list using LINQ. How can I do this?
-
Sedat Kapanoglu over 12 yearsTo elaborate on the second approach, it's the functional approach which treats modified versions of lists as new instances. This allows list immutability and has certain inherent advantages in functional programming model. It may not be as performant though depending on the scenario. For further reference: en.wikipedia.org/wiki/Immutable_object
-
Maxim Gershkovich over 10 yearsThere are kinda exceptions to this. For example needing to append an empty ListItem to a collection for the purposes of display. In this scenario you can accomplish this with a Union as such StockCollection.Union(new[] { new Stock() { Name = "" } }). While this is certainly not a great practice sometimes its useful.
-
Maxim Gershkovich over 10 yearsI've used it like this in MVC @Html.DropDownListFor(o => o.StockID, Model.Stock.Union(new[] { new Stock() { Name = "" } }).Select(o => new SelectListItem() { Text = o.Name, Value = o.StockID.ToString() })
-
Jon Skeet over 10 years@MaximGershkovich: That isn't appending a list item to a collection. It's creating a new sequence out of two sequences. (Personally I'd use
Concat
rather thanUnion
, but that's a different matter.) "Adding an item to a collection" necessarily changes the contents of the collection - you're not doing that. -
Maxim Gershkovich over 10 yearsFair enough but I feel like that's arguing about semantics rather than the actual intent of the OP.
-
Jon Skeet over 10 years@Maxim: the intent of the OP seems to be adding to a collection, given that that's what was asked for. Union simply doesn't do that.