How to add Flutter DropdownButtonFormField
Solution 1
The widget does exist in flutter/materials.dart
.
DropdownButtonFormField
requires the items
property to be defined in its constructor. You need to use it like so:
import 'package:flutter/material.dart';
...
@override
Widget build(BuildContext context) {
return Column(children: <Widget>[
DropdownButtonFormField(
items: <DropdownMenuItem>[
// Put widgets in the drop down menu here
],
)
]);
}
Solution 2
The widget does exist in flutter/materials.dart.
import 'package:flutter/material.dart';
...
String _selectedValue;
List<String> listOfValue = ['1', '2', '3', '4', '5'];
@override
Widget build(BuildContext context) {
return DropdownButtonFormField(
value: _selectedValue,
hint: Text(
'choose one',
),
isExpanded: true,
onChanged: (value) {
setState(() {
_selectedValue = value;
});
},
onSaved: (value) {
setState(() {
_selectedValue = value;
});
},
validator: (String value) {
if (value.isEmpty) {
return "can't empty";
} else {
return null;
}
},
items: listOfValue
.map((String val) {
return DropdownMenuItem(
value: val,
child: Text(
val,
),
);
}).toList(),
);
}
Related videos on Youtube
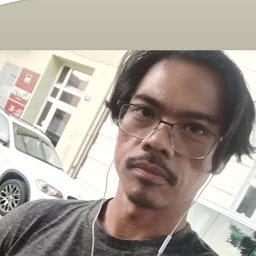
Cyrus Talladen
Updated on July 01, 2022Comments
-
Cyrus Talladen almost 2 years
Beginner Flutter enthusiast here, just learning the widget system. Wanted to implement autocomplete textfield using out of the box widgets (not plugins ok) The DropdownButtonFormField is perfect for my use case but when I try using it, the compiler gives me a Method not found error.
Compiler message: lib/expanding_text.dart:100:11: Error: Method not found: 'DropdownButtonFormField'. DropdownButtonFormField(), ^^^^^^^^^^^^^^^^^^^^^^^ lib/expanding_text.dart:100:11: Error: The method 'DropdownButtonFormField' isn't defined for the class '#lib1::_TripItemState'. Try correcting the name to the name of an existing method, or defining a method named 'DropdownButtonFormField'. DropdownButtonFormField(),
Heres my code (the relevant part)
import 'package:flutter/material.dart'; ... @override Widget build(BuildContext context) { return Column( children: <Widget>[ DropdownButtonFormField<String>( items: [DropdownMenuItem<String>(child:Text("test"))], ),
Looking at the docs it seems like I can freely add it to the widget tree without extra configuration. But obviously I'm missing something here because of the error.
So to troubleshoot whats happening, is DropdownButtonFormField still in the material library?
Is there anything else Im missing?
-
Cyrus Talladen about 5 yearsthanks I actually have that on the original code. edited the post.