How to add JavaFX runtime to Eclipse in Java 11?
Solution 1
Following the getting started guide, these are the required steps to run JavaFX 11 from Eclipse.
-
Install Eclipse 2018-09 from here.
-
Install JDK 11 from here.
-
Add Java 11 as an installed JRE to Eclipse: Eclipse -> Window -> Preferences -> Java -> Installed JREs -> Add.
-
Download JavaFX 11 ea from here.
-
Create a User Library: Eclipse -> Window -> Preferences -> Java -> Build Path -> User Libraries -> New. Name it JavaFX11 and include the jars under the lib folder from JavaFX 11-ea.
-
Create a Java project. You don't need to add a module-path class. Make sure that you select Java 11 and you add the JavaFX11 library to the project's modulepath.
-
Add a package
javafx11
and the main application classHelloFX
:
package javafx11;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class HelloFX extends Application {
@Override
public void start(Stage stage) {
String version = System.getProperty("java.version");
Label l = new Label ("Hello, JavaFX 11, running on "+version);
Scene scene = new Scene (new StackPane(l), 300, 200);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch();
}
}
Note that the editor shouldn't complain about JavaFX classes, as we have included the user library.
-
Add runtime arguments. Edit the project's run configuration, and add these VM arguments:
--module-path C:\Users<user>\Downloads\javafx-sdk-11\lib --add-modules=javafx.controls
-
Finally, run the project. It should work fine.
Solution 2
I'd like to share my findings regarding launching a javafx 11 app. I'm talking about an existing legacy javafx app originally developed without modules (e.g. w/ Java 8).
The most interesting way IMO is Method #2b below, which requires the minimum of changes, i.e. it doesn't need -module-path
argument. I mention that I'm using maven, so cf. previous answers the first step is to add a dependency in your pom.xml
towards javafx. E.g.:
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>11.0.2</version>
</dependency>
In order to launch, I found several options:
1a) Launch using maven from command line
Configure in your pom.xml
a section like:
<build>
<plugins>
...
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>1.6.0</version>
<executions>
<execution>
<goals>
<goal>java</goal>
</goals>
</execution>
</executions>
<configuration>
<mainClass>org.openjfx.hellofx.MainApp</mainClass>
</configuration>
</plugin>
</plugins>
</build>
After this, from a console window you can run mvn exec:java
, and this should launch the application. Maven will take care of adding all the entries from classpath to module path. I remind the CTRL + ALT + T
shortcut in Eclipse, that opens a terminal window directly in the IDE.
1b) Launch using maven, but as an Eclipse launch config
Right click on the project > Run As > Maven Build.... Then enter exec:java
in the Goals text box. Advantage over the previous method: a bit more integrated to Eclipse. And easy debugging. You only need to relaunch the launch config in Debug mode and that's it. May I remind that the Eclipse launch configs can be stored as .launch
files directly in the project dir (repo), thus shareable/reusable by colleagues. Use the last tab, Common, in the Edit Configuration window.
2a) Launch using Eclipse and specifying -module-path
using a variable
Right click on the main class > Run As > Java Application. The first time you click a new launch configuration is created; probably it won't work, so you need to edit it (e.g. **Run button (w/ Play icon) in the toolbar > Run configurations > select the one corresponding to your class. Or CTRL + click
on it after clicking on Play).
Then add this in Arguments > VM arguments:
--module-path ${project_classpath:REPLACE_ME_WITH_YOUR_PROJECT_NAME} --add-modules javafx.controls,javafx.fxml
So the trick here was to use the ${project_classpath}
variable. Because otherwise you should have needed to write exactly the path towards the javafx jar, that are somewhere in your .m2
repo. Doing this would make the launch config not easily reusable by colleagues.
2b) Launch using Eclipse WITHOUT specifying -module-path
This is la piece de résistence, which I found by mistake, after about 5h of Java 11 & modules "fun". Your application can work out of the box, without touching -module-path
. The javafx libs will of course still need to be in your classpath (but this is handled by mvn). The trick is (cf. this) that your main app SHOULD NOT extend Application
(e.g. MyApplication
below). If this is your case, then make a new class with a main()
function that does e.g.:
Application.launch(MyApplication.class);
NOTE
In my case (i.e. on Windows, having a default JDK 8 in the system), there is a known issue regarding launching javafx. The solution is to add the following VM arg: -Djava.library.path=C:
. So actually for all 4 methods described above I needed to add this.
Solution 3
I had problems regarding FXMLLoader
... It was not able to find the class.
I resolved this by changing the arguments showed above, to load all modules, not only javafx.controls
:
--module-path <path to the javafx sdk> --add-modules=ALL-MODULE-PATH
Solution 4
As mentioned by @Cristian, creating a separate Main class, seems to do the trick, without any other arguments.
public class Main {
public static void main(String[] args) {
Application.launch(MainWindow.class);
}
}
public class MainWindow extends Application {
@Override
public void start(Stage stage) {
String javaVersion = System.getProperty("java.version");
String javafxVersion = System.getProperty("javafx.version");
Label l = new Label("Hello, JavaFX " + javafxVersion + ", running on Java " + javaVersion + ".");
Scene scene = new Scene(new StackPane(l), 640, 480);
stage.setScene(scene);
stage.show();
}
// do not use this method
// public static void main(String[] args) {
// Application.launch(MainWindow.class);
// }
}
Solution 5
@José Pereda is perfectly right, everything works as it should. I have tested it with the Eclipse release 2018-12. So, after you download the IDE (https://www.eclipse.org/downloads/packages/release/2018-12/r/eclipse-ide-java-developers) and install the e(fx)clipse plugin, you should be able to create a "JavaFx Project" item in your workspace.
The next step is to delete the "JavaFX SDK" from the "Classpath" module (Properties -> Java Build Path -> Libraries) and add the Maven dependency in order to remove compile errors:
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>11.0.1</version>
</dependency>
The last step is to add a VM argument to "Run Configuration" of your JavaFX class for starting the application (Run Configurations -> Arguments tab -> VM arguments):
--module-path C:\path to the OpenJFX\javafx-sdk-11.0.1\lib --add-modules=javafx.controls
and it should work after you run it. Of course, you should download the JavaFX SDK before that (https://gluonhq.com/products/javafx/), unzip it and use the path to "lib" folder in the aforementioned VM argument.
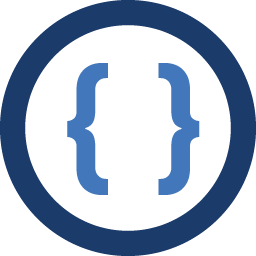
Admin
Updated on August 19, 2020Comments
-
Admin over 3 years
I am getting the following error as Java 11 excluded the JavaFX as part of the latest version.
Error: JavaFX runtime components are missing, and are required to run this application
So how can I add JavaFX to Eclipse in Java 11? Thanks.
-
Admin over 5 yearsDone. i Have one more query how we can avoid giving module path in VM arguments, is there any way i can read the modules from repository ?
-
José Pereda over 5 yearsFor that you can use Maven or Gradle, where the JavaFX dependencies are managed automatically via a local repo. See the mentioned guide for a Maven setup.
-
c0der over 5 yearsCould you explain " Install JDK 11 from here" ? The link points to a .zip file. Do you mean just unzip it and place it somewhere it can be used for step 3 ?
-
José Pereda over 5 yearsYou could install Oracle JDK 11, or you can install Open JDK 11 from that link, without an installer, so just unzip it to your preferred location.
-
carlao2005 about 5 yearsMaybe the good people of this post could take a look at this: stackoverflow.com/questions/55689045/… Best regards!
-
shawn1874 over 4 yearsWhy should one have to manually download an entire Java Fx SDK when dependency management is supposed to be the job of Maven?
-
Andrew S over 4 yearsJosé, what do you mean by "include the jars under the lib folder from JavaFX 11-ea."?
-
José Pereda over 4 years@AndrewS See openjfx.io/openjfx-docs/#IDE-Eclipse, once you download the JavaFX SDK there is a
lib
folder with the jars for each module:javafx.base.jar
, ... -
Andrew S over 4 yearsAh I see that now. I have done it. I'm also going to try to installed e(fx)clipse to see if some things can be automated though the wizzards as they once were with Java 8. For me it says "The import javafx cannot be resolved".
-
Andrew S over 4 yearsWhy do we have to add a package called JavaFX11 for our Main.java. It seems quite restrictive?
-
Andrew S over 4 yearsWhere should Application.launch(MyApplication.class); go? I think you need to give an example of the entire file.
-
GeoffL about 4 years@AndrewS It's just an example package name, you can call it anything.
-
3142 maple about 4 yearsJust a reminder, if your JavaFX path has space in it, you should wrap it in quotes when you use it in runtime arguments. I just encountered that problem.
-
Patter over 3 years2b) is a really nice observation which saved me a lot of time - thanks!
-
Tillmann about 2 years2b) works for me also, but it produces this warning:
Unsupported JavaFX configuration: classes were loaded from 'unnamed module @eef29c6'