How to add marker in the google map using flutter?
8,245
Make sure you add your API_KEY
. A working example of your requirement follows
import 'package:flutter/material.dart';
import 'package:google_maps_flutter/google_maps_flutter.dart';
import 'package:http/http.dart' as http;
import 'dart:convert';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
LatLng latlng = LatLng(
-33.8670522,
151.1957362,
);
Iterable markers = [];
@override
void initState() {
super.initState();
getData();
}
getData() async {
try {
final response =
await http.get('https://maps.googleapis.com/maps/api/place/nearbysearch/json?location=-33.8670522,151.1957362&radius=1500&type=restaurant&key=API_KEY');
final int statusCode = response.statusCode;
if (statusCode == 201 || statusCode == 200) {
Map responseBody = json.decode(response.body);
List results = responseBody["results"];
Iterable _markers = Iterable.generate(10, (index) {
Map result = results[index];
Map location = result["geometry"]["location"];
LatLng latLngMarker = LatLng(location["lat"], location["lng"]);
return Marker(markerId: MarkerId("marker$index"),position: latLngMarker);
});
setState(() {
markers = _markers;
});
} else {
throw Exception('Error');
}
} catch(e) {
print(e.toString());
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: GoogleMap(
markers: Set.from(
markers,
),
initialCameraPosition: CameraPosition(target: latlng, zoom: 15.0),
mapType: MapType.hybrid,
onMapCreated: (GoogleMapController controller) {},
),
);
}
}
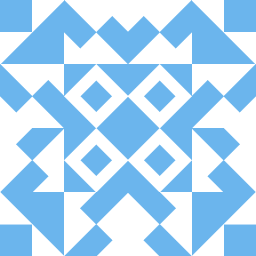
Author by
Vipin Malik
Front End Developer with knowledge about Html5, CSS3, JavaScript, Bootstrap 4 and few of the Back end Technologies like MySql, Php. Also, I am a new Flutter Developer with Knowledge about dart language, C, C++.
Updated on December 12, 2022Comments
-
Vipin Malik 5 months
I am creating a nearby flutter app which shows restaurant around your location. i have found the nearby location but unable to add markers on the nearby coordinates.I want to know how to add markers to my location using google API and how to load the location from the list to my map.
void getData() async { http.Response response = await http.get( 'https://maps.googleapis.com/maps/api/place/nearbysearch/json?location=-33.8670522,151.1957362&radius=1500&type=restaurant&key=API_KEY'); if (response.statusCode == 200) { String data = response.body; var decodedData = jsonDecode(data); print(decodedData); List<String> names = []; List<double> lat = []; List<double> lng = []; for (var i = 0; i < 10; i++) { names.add(decodedData['results'][i]['name']); lat.add(decodedData['results'][i]['geometry']['location']['lat']); lng.add(decodedData['results'][i]['geometry']['location']['lng']); } print(names); print(lat); print(lng); } } Expanded( child: Container( height: MediaQuery.of(context).size.height, width: MediaQuery.of(context).size.width, child: GoogleMap( initialCameraPosition: CameraPosition( target: LatLng(-33.8670522, 151.1957362), zoom: 14.4746, ), markers: Set<Marker>.of(markers.values), onMapCreated: (GoogleMapController controller) { _controller.complete(controller); }, ), ), ),
-
Vipin Malik almost 4 yearsWorks like a charm, BTW I have one more issue relating to the same app so If you like, go check it out please. Thanks for the answer.
-
Erdinç Çorbacı over 3 yearsGreat ! Love this type of answers :),clean and fully explaining
-
Muhammad Dyas Yaskur about 3 yearsWhile this code may solve the question, including an explanation really helps to improve the quality of your post. Remember that you are answering the question for readers in the future, and those people might not know the reasons for your code suggestion