How to add minutes to current time in swift
Solution 1
Two approaches:
-
Use
Calendar
anddate(byAdding:to:wrappingComponents:)
. E.g., in Swift 3 and later:let calendar = Calendar.current let date = calendar.date(byAdding: .minute, value: 5, to: startDate)
-
Just use
+
operator (see+(_:_:)
) to add aTimeInterval
(i.e. a certain number of seconds). E.g. to add five minutes, you can:let date = startDate + 5 * 60
(Note, the order is specific here: The date on the left side of the
+
and the seconds on the right side.)You can also use
addingTimeInterval
, if you’d prefer:let date = startDate.addingTimeInterval(5 * 60)
Bottom line, +
/addingTimeInterval
is easiest for simple scenarios, but if you ever want to add larger units (e.g., days, months, etc.), you would likely want to use the calendrical calculations because those adjust for daylight savings, whereas addingTimeInterval
doesn’t.
For Swift 2 renditions, see the previous revision of this answer.
Solution 2
You can use Calendar's method
func date(byAdding component: Calendar.Component, value: Int, to date: Date, wrappingComponents: Bool = default) -> Date?
to add any Calendar.Component
to any Date
. You can create a Date extension to add x minutes to your UIDatePicker
's date:
Xcode 8 and Xcode 9 • Swift 3.0 and Swift 4.0
extension Date {
func adding(minutes: Int) -> Date {
return Calendar.current.date(byAdding: .minute, value: minutes, to: self)!
}
}
Then you can just use the extension method to add minutes to the sender (UIDatePicker):
let section1 = sender.date.adding(minutes: 5)
let section2 = sender.date.adding(minutes: 10)
Playground testing:
Date().adding(minutes: 10) // "Jun 14, 2016, 5:31 PM"
Solution 3
Swift 4:
// add 5 minutes to date
let date = startDate.addingTimeInterval(TimeInterval(5.0 * 60.0))
// subtract 5 minutes from date
let date = startDate.addingTimeInterval(TimeInterval(-5.0 * 60.0))
Swift 5.1:
// subtract 5 minutes from date
transportationFromDate.addTimeInterval(TimeInterval(-5.0 * 60.0))
Solution 4
You can use in swift 4 or 5
let date = Date()
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "yyyy-MM-dd H:mm:ss"
let current_date_time = dateFormatter.string(from: date)
print("before add time-->",current_date_time)
//adding 5 miniuts
let addminutes = date.addingTimeInterval(5*60)
dateFormatter.dateFormat = "yyyy-MM-dd H:mm:ss"
let after_add_time = dateFormatter.string(from: addminutes)
print("after add time-->",after_add_time)
output:
before add time--> 2020-02-18 10:38:15
after add time--> 2020-02-18 10:43:15
Solution 5
extension Date {
func withAddedMinutes(minutes: Double) -> Date {
addingTimeInterval(minutes * 60)
}
func withAddedHours(hours: Double) -> Date {
withAddedMinutes(minutes: hours * 60)
}
}
useCase
let anHourFromNow = Date().withAddedHours(hours: 1)
let aMinuteFromNow = Date().withAddedMinutes(minutes: 1)
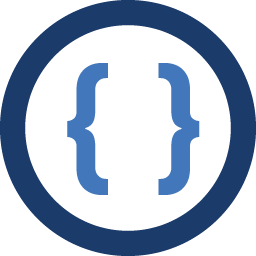
Admin
Updated on April 30, 2021Comments
-
Admin about 3 years
I am new to Swift and am trying a scheduler. I have the start time selected and I need to add 5 minutes (or multiples of it) to the start time and display it in an
UILabel
?@IBAction func timePickerClicked(sender: UIDatePicker) { var dateFormatter = NSDateFormatter() dateFormatter.timeStyle = NSDateFormatterStyle.ShortStyle var dateStr = dateFormatter.stringFromDate(startTime.date) let sttime = dateStr startTimeDisplay.text = dateStr } // How to advance time by 5 minutes for each section based on the start time selected and display time // section 1 = start time + 5 // section 2 = start time + 10*
-
Declan McKenna over 6 yearsUnder what circumstances does
.date(byAdding:value:to)
returnnil
? Are we safe to force unwrap here? -
Leo Dabus over 6 years@Deco How would adding minutes to a Date return nil? If the world doesn't come to an end in the resulting date you are safe :)
-
Declan McKenna over 6 yearsThe documentation isn't clear on how this can return nil. This question explains it, you're right it's completely safe here. stackoverflow.com/questions/39358131/…
-
KlimczakM almost 6 yearsUnfortunately, months and years are incorrect. 7 * 4 * 12 = 336, this is much less than 365.
-
KlimczakM almost 6 yearsIt can be improved by calculating months like this:
self.days * 30
and years:self.days * 365
. This isn't perfect because the year can have 366 days, and month can be different than 30 days, but it is still better than 28/336. -
Steven Stefanik over 5 yearsYou should definitely not use this for all the reasons listed above, it's inaccurate in a bunch of different cases. Use the built-in data arithmetic functions
-
Nishant over 4 yearsWhich of these 2 approaches is more performant? addingTimeInterval or calendar.date(byAdding...
-
Rob over 4 yearsThe
addingTimeInterval
is undoubtedly more performant (though likely not material unless you’re doing this millions of times). What is more critical, though, is if you’re talking about units of measure in days (or longer), then the calendrical rendition is preferable because it will do all of the daylight savings adjustments for you. -
Mehdi Chennoufi over 4 yearsMonths are not always equal to 4 weeks, also how about leap years with this ?