How to add "provided" dependencies back to run/test tasks' classpath?
Solution 1
For a similar case I used in assembly.sbt:
run in Compile <<= Defaults.runTask(fullClasspath in Compile, mainClass in (Compile, run), runner in (Compile, run))
and now the 'run' task uses all the libraries, including the ones marked with "provided". No further change was necessary.
Update:
@rob solution seems to be the only one working on latest SBT version, just add to settings
in build.sbt
:
run in Compile := Defaults.runTask(fullClasspath in Compile, mainClass in (Compile, run), runner in (Compile, run)).evaluated,
runMain in Compile := Defaults.runMainTask(fullClasspath in Compile, runner in(Compile, run)).evaluated
Solution 2
Adding to @douglaz' answer,
runMain in Compile <<= Defaults.runMainTask(fullClasspath in Compile, runner in (Compile, run))
is the corresponding fix for the runMain task.
Solution 3
Another option is to create separate sbt projects for assembly vs run/test. This allows you to run sbt assemblyProj/assembly
to build a fat jar for deploying with spark-submit, as well as sbt runTestProj/run
for running directly via sbt with Spark embedded. As added benefits, runTestProj will work without modification in IntelliJ, and a separate main class can be defined for each project in order to e.g. specify the spark master in code when running with sbt.
val sparkDep = "org.apache.spark" %% "spark-core" % sparkVersion
val commonSettings = Seq(
name := "Project",
libraryDependencies ++= Seq(...) // Common deps
)
// Project for running via spark-submit
lazy val assemblyProj = (project in file("proj-dir"))
.settings(
commonSettings,
assembly / mainClass := Some("com.example.Main"),
libraryDependencies += sparkDep % "provided"
)
// Project for running via sbt with embedded spark
lazy val runTestProj = (project in file("proj-dir"))
.settings(
// Projects' target dirs can't overlap
target := target.value.toPath.resolveSibling("target-runtest").toFile,
commonSettings,
// If separate main file needed, e.g. for specifying spark master in code
Compile / run / mainClass := Some("com.example.RunMain"),
libraryDependencies += sparkDep
)
Solution 4
If you use sbt-revolver
plugin, here is a solution for its "reStart" task:
fullClasspath in Revolver.reStart <<= fullClasspath in Compile
UPD: for sbt-1.0 you may use the new assignment form:
fullClasspath in Revolver.reStart := (fullClasspath in Compile).value
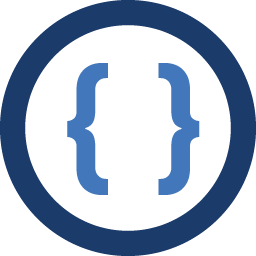
Admin
Updated on July 03, 2022Comments
-
Admin almost 2 years
Here's an example
build.sbt
:import AssemblyKeys._ assemblySettings buildInfoSettings net.virtualvoid.sbt.graph.Plugin.graphSettings name := "scala-app-template" version := "0.1" scalaVersion := "2.9.3" val FunnyRuntime = config("funnyruntime") extend(Compile) libraryDependencies += "org.spark-project" %% "spark-core" % "0.7.3" % "provided" sourceGenerators in Compile <+= buildInfo buildInfoPackage := "com.psnively" buildInfoKeys := Seq[BuildInfoKey](name, version, scalaVersion, target) assembleArtifact in packageScala := false val root = project.in(file(".")). configs(FunnyRuntime). settings(inConfig(FunnyRuntime)(Classpaths.configSettings ++ baseAssemblySettings ++ Seq( libraryDependencies += "org.spark-project" %% "spark-core" % "0.7.3" % "funnyruntime" )): _*)
The goal is to have spark-core
"provided"
so it and its dependencies are not included in the assembly artifact, but to reinclude them on the runtime classpath for therun
- andtest
-related tasks.It seems that using a custom scope will ultimately be helpful, but I'm stymied on how to actually cause the default/global run/test tasks to use the custom
libraryDependencies
and hopefully override the default. I've tried things including:(run in Global) := (run in FunnyRuntime)
and the like to no avail.
To summarize: this feels essentially a generalization of the web case, where the servlet-api is in "provided" scope, and run/test tasks generally fork a servlet container that really does provide the servlet-api to the running code. The only difference here is that I'm not forking off a separate JVM/environment; I just want to manually augment those tasks' classpaths, effectively "undoing" the "provided" scope, but in a way that continues to exclude the dependency from the assembly artifact.