How to add sound files to your bundle in Xcode?
Solution 1
This answer is top of Google searching for 'How to add sound files to your bundle in Xcode?', so to help rookies like me, here is step by step how you add sounds to your bundle in Xcode. (Short answer, just drag them into the sidebar and check the 'Copy items if needed')
Put the sounds in a folder (you don't have to but keeps them organized)
When you drag the folder in, it will give you a few options. Make sure 'Copy items if needed' is checked. It should be default.
You are done! Access your sound by getting the path and URL based on the file name.
Like this:
func playSaveSound(){
let path = Bundle.main.path(forResource: "upward.wav", ofType: nil)!
let url = URL(fileURLWithPath: path)
do {
//create your audioPlayer in your parent class as a property
audioPlayer = try AVAudioPlayer(contentsOf: url)
audioPlayer.play()
} catch {
print("couldn't load the file")
}
}
This article has a nice little overview of how to play sounds.
Solution 2
There is an extra step here that XCode is doing implicitly, and it's possible to have your stuff configured in such a way that it doesn't get done. You need to make sure that the wav file is in the list of items under your target's Build Phases -> Copy Bundle Resources setting.
If you just have a main target for your app and nothing fancy, then this should be done automatically when you add the file to your folder in XCode. But I have a screwy setup where it's not, and I had to dig to find it.
Solution 3
Follow these steps:
- add the sound files in your project by dragging them to the Xcode sidebar
- a dialog will appear - check on
Copy items needed
and add files. - to test if files were added properly, in Xcode right-click on any of the added files and click on
show in Finder
. If the file exists then on runtime it will not throw an error.
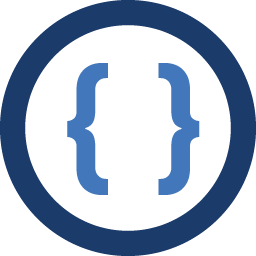
Admin
Updated on June 06, 2022Comments
-
Admin almost 2 years
I am trying to make an application with sound. I have asked a question before how to add sound to an app in Xcode but every time I run the app, the console throws this error,
MagicSound.wav does not exist in main bundle
when writing my code I basically told the program to crash the application and writeMagicSound.wav does not exist in main bundle
if it comes to be true. My question is how do you add a file (specifically a sound file) to your bundle in Xcode. I always assumed putting a file in your assets is the same thing as putting it in your bundle.Here is my code,
import UIKit import AVFoundation class ViewController: UIViewController { var magicSound: AVAudioPlayer = AVAudioPlayer() @IBOutlet var Answer: UILabel! var AnswerArray = ["Yes", "No", "Maybe", "Try Again", "Not Now", "No Doubt", "Yes Indeed", "Of course", "Definetley Not"] var chosenAnswer = 0 override func viewDidLoad() { super.viewDidLoad() // Do any additional setup after loading the view, typically from a nib. if let magicFile = Bundle.main.path(forResource: "MagicSound", ofType: "wav") { _ = try? AVAudioPlayer(contentsOf: URL (fileURLWithPath: magicFile)) } else { print( "MagicSound.wav does not exist in main bundle" ) } } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() // Dispose of any resources that can be recreated. } override func motionEnded(_ motion: UIEventSubtype, with event: UIEvent?) { if event?.subtype == motion { printAnswer() randomAnswer() animation() showingAnswerAnimation() magicSound.play() } }
If anybody could help that would be great!
Thanks.
-
Chris Gong about 5 yearsThe key for me here was selecting
Create groups
instead ofCreate folder references
in step 3, thanks a ton!