How to align a <div> to the middle (horizontally/width) of the page
Solution 1
<body>
<div style="width:800px; margin:0 auto;">
centered content
</div>
</body>
Solution 2
position: absolute
and then top:50%
and left:50%
places the top edge at the vertical center of the screen, and the left edge at the horizontal center, then by adding margin-top
to the negative of the height of the div, i.e., -100 shifts it above by 100 and similarly for margin-left
. This gets the div
exactly in the center of the page.
#outPopUp {
position: absolute;
width: 300px;
height: 200px;
z-index: 15;
top: 50%;
left: 50%;
margin: -100px 0 0 -150px;
background: red;
}
<div id="outPopUp"></div>
Solution 3
Flexbox solution is the way to go in/from 2015. justify-content: center
is used for the parent element to align the content to the center of it.
HTML
<div class="container">
<div class="center">Center</div>
</div>
CSS
.container {
display: flex;
justify-content: center;
}
Output
.container {
display: flex;
justify-content: center;
}
.center {
width: 400px;
padding: 10px;
background: #5F85DB;
color: #fff;
font-weight: bold;
font-family: Tahoma;
}
<div class="container">
<div class="center">Centered div with left aligned text.</div>
</div>
Solution 4
Do you mean that you want to center it vertically or horizontally? You said you specified the
height
to 800 pixels, and wanted the div not to stretch when thewidth
was greater than that...To center horizontally, you can use the
margin: auto;
attribute in CSS. Also, you'll have to make sure that thebody
andhtml
elements don't have any margin or padding:
html, body { margin: 0; padding: 0; }
#centeredDiv { margin-right: auto; margin-left: auto; width: 800px; }
Solution 5
<div></div>
div {
display: table;
margin-right: auto;
margin-left: auto;
}
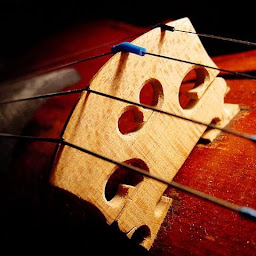
Shimmy Weitzhandler
Updated on September 12, 2021Comments
-
Shimmy Weitzhandler over 2 years
I have a
div
tag withwidth
set to 800 pixels. When the browser width is greater than 800 pixels, it shouldn't stretch thediv
, but it should bring it to the middle of the page. -
gonzohunter almost 15 yearsThis is correct for demo purposes, but obviously not using inline styles in the final markup
-
bartosz.r over 12 yearsbe sure to check HTML mode for IE6 or 7. If you use anything other than 4.01 strict you may have problems. Most of the time text-align works as avdgaag says.
-
bartosz.r over 12 yearsOr go out from quircks mode and use a strict mode, it helps a lot, when you want to use features like hover, auto-margins and many others.
-
Manse about 12 yearsThe
<center>
tag was deprecated in html 4 -
surfmuggle over 11 yearsI tried it out: jsfiddle.net/nqEar/show On my monitor (1920px) on Chrome 22 it is not centered.
-
Marcus over 10 yearsThx, your answer is the one and only cross-browser solution, it should be accepted...worth mentioning that it also works with "position:relative" if you have other divs on top and below (in this case only "left:50%" and "margin:0px -150px;" are important).
-
ʍǝɥʇɐɯ almost 10 yearsposition: fixed worked for me and might work best for anyone else where the added div is in some tree of absolute/relative divs already.
-
Chris Aplin over 9 yearsPS, this is margin: 0 auto;
-
Bill Masters over 8 yearsIt may have been depreciated, but it's still the simplest solution and works on all browsers.
-
iMatoria over 8 yearsThis could be in percentage as well.
width:90%;left:50%;margin-left:45%;
-
jkdev over 8 years@rybo111 Then you don't need to. The idea is that 'left' is the default for text-align, and if it isn't restored then the entire div will inherit 'text-align: center'.
-
themhz over 8 yearswhy do you use width:800px ? will this work for all screens?
-
AgileJon over 8 years@themis - I only used 800px because the original question wanted that as the max width.
-
Taylor Brown about 8 years@ChrisAplin This works for me fine as is. Did you downvote because of this? Not necessary. Someone downvoted this for no apparent reason this is working fine for me I use it everywhere.
-
Alex Parij over 7 years"position: fixed" works better
-
Mohammad Usman over 7 years@BillMasters May be it is working now but at some time in future it will become obsolete.
-
dresh over 7 yearsthis is what i needed. thanks. using the more upvoted answers would only help to position a popup. this answer helps position any div in the center horizontally.
-
Kamal Soni about 7 yearsThis answer is useful when you don't want to set the width to a fix pixels of 800px. The size can be 80% and it will cover 80% of the screen size available, which seems more dynamic.
-
JRichardsz about 7 years.divClass works without width:300px; Thanks!!!
-
Shreyan Mehta almost 7 yearsnot working...:/
-
Billu almost 7 yearsI recommend Andrei's solution. Working fine in center with all aspects.
-
est over 6 years@MohammadUsman I think this tag would survive longer than some fancy ES6 module npm dependency shit.
-
mwag over 6 yearsusing
transform: translateX(-50%)
is more versatile than using a negative margin as a way to account for the div's width. same applies for translateY and height -
Hassan Baig about 6 years@gonzohunter: why not?
-
Luciano Andress Martini about 6 yearsnot working here too.. it is doing nothing... the text is still on the top of the page... =[
-
Jerry Dodge about 6 yearsWorks fine here. I used
max-width
instead of justwidth
. Allows it to scale down. "the text is still on the top of the page..." This is not related to vertical alignment. It's related to horizontal alignment. Also, why are we talking about IE 6-7 in 2011? IE7 was already on its downfall. Why are we talking about IE at all? Do people still use IE these days? -
dawn almost 6 years<center> is the savior we all need.
-
Peter Mortensen about 5 yearsThe second link is effectively broken ("This Web page is parked for FREE...").
-
Wilt about 5 years@PeterMortensen I improved my answer by replacing the link with a new one with similar solution and by adding the code to the answer to prevent such problems in the future (link only answers are no good). Also removed the link to the first solution because it wasn't that great actually.
-
Joseph about 5 yearsThis worked for me. It also works when you do not specify width of div in css and when you also decide to specify the width in css. Thank you.
-
Wrong almost 5 yearsthis aligns the text into the div too
-
Shayan over 4 yearsDidn't work for my
div
which has atable
in it. -
A. Kali over 4 yearsFunny this is the simplest answer and the only one that is actually correct. Should get more votes.
-
MattSom about 4 years@mwag
transform: translate
blurs the content for me. I'm trying to find something alternative for that actually. -
mwag about 4 years@MattSom have you tried adjusting anti-aliasing e.g. stackoverflow.com/questions/6411361/…?
-
johannchopin about 4 yearsAs you can see it's enable highlights for
css
-
CodingEE about 4 yearsThis CSS has to be the best way to do it. You can set the width 80% here, and then in the inner-div, set it to 100%. And this way, it will scale correctly with the browser resizing. I love it. Thank you very much.
-
Admin almost 4 yearsThis should be the accepted answer....
-
Arun Ramachandran almost 4 yearsI would say it's a neater and elegant solution!
-
Feng Zhang over 3 years<div style="text-align: center;">
-
Vicky Gupta about 3 yearsthis worked best for me, easiest and the most straight forward way to align div in center of page