How to animate gridview.builder in Flutter?
4,306
use auto_animated,
Widget buildAnimatedItem(
BuildContext context,
int index,
Animation<double> animation,
) {
var color = colorsList[index];
return FadeTransition(
opacity: Tween<double>(
begin: 0,
end: 1,
).animate(animation),
child: SlideTransition(
position: Tween<Offset>(
begin: Offset(0, -0.1),
end: Offset.zero,
).animate(animation),
child: MaterialButton(
elevation: 0,
color: color,
shape: CircleBorder(),
onPressed: () {
Navigator.pop(context);
setState(() {
AnimatedList(
itemBuilder: (context, index,
animation) {
return SlideTransition(
position: animation
.drive(List<color>));
},
);
mainColor = color;
});
}),
),
);
}
LiveGrid.options(
options: options,
itemBuilder: buildAnimatedItem,
itemCount: itemsCount,
gridDelegate: SliverGridDelegateWithFixedCrossAxisCount(
childAspectRatio: 1.0,
crossAxisSpacing: 5.0,
mainAxisSpacing: 5.0,
crossAxisCount: 5),
);
OR
this might help, flutter_staggered_animations:
@override
Widget build(BuildContext context) {
int columnCount = 3;
return Scaffold(
body: AnimationLimiter(
child: GridView.count(
crossAxisCount: columnCount,
children: List.generate(
100,
(int index) {
return AnimationConfiguration.staggeredGrid(
position: index,
duration: const Duration(milliseconds: 375),
columnCount: columnCount,
child: ScaleAnimation(
child: FadeInAnimation(
child: YourListChild(),
),
),
);
},
),
),
),
);
}
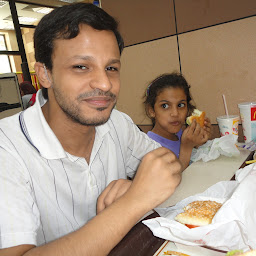
Author by
Ahmed Loutfy
Updated on December 31, 2022Comments
-
Ahmed Loutfy over 1 year
I'm new to Flutter and Dart and still learning them.
I need to make animation to the coloured circles grid as in the following gif:
I searched a lot online with no result.
Here is the code that I'm working on:
child: GridView.builder( padding: const EdgeInsets.all(5.0), shrinkWrap: true, itemCount: colorsList.length, gridDelegate: SliverGridDelegateWithFixedCrossAxisCount( childAspectRatio: 1.0, crossAxisSpacing: 5.0, mainAxisSpacing: 5.0, crossAxisCount: 5), itemBuilder: (context, index) { var color = colorsList[index]; return MaterialButton( elevation: 0, color: color, shape: CircleBorder(), onPressed: () { Navigator.pop(context); setState(() { AnimatedList( itemBuilder: (context, index, animation) { return SlideTransition( position: animation .drive(List<color>)); }, ); mainColor = color; }); }); }, ),
Appreciate any help
-
Ahmed Loutfy almost 3 yearsSorry for the late reply, can you please embed the code into my code? I tried many times, and every time the output appears distorted.
-
Jim almost 3 yearsupdated with another option (combined your code)
-
Ahmed Loutfy almost 3 yearsThe console throwed an error: ======== Exception caught by widgets library ======================================================= The following NoSuchMethodError was thrown building NewCounter(dirty, state: _NewCounterState#ba01e): Class '_NewCounterState' has no instance method 'build' with matching arguments. Receiver: Instance of '_NewCounterState' Tried calling: build(Instance of 'StatefulElement') Found: build(BuildContext) => Widget
-
Ahmed Loutfy almost 3 yearshere is the full code inside new_counter.dart justpaste.it/5xacp. Help me!