How to assert elements contains text in Selenium using JUnit
Solution 1
Use:
String actualString = driver.findElement(By.xpath("xpath")).getText();
assertTrue(actualString.contains("specific text"));
You can also use the following approach, using assertEquals
:
String s = "PREFIXspecific text";
assertEquals("specific text", s.substring(s.length()-"specific text".length()));
to ignore the unwanted prefix from the string.
Solution 2
Two Methods assertEquals and assertTrue could be used. Here is the usage
String actualString = driver.findElement(By.xpath("xpath")).getText();
String expectedString = "ExpectedString";
assertTrue(actualString.contains(expectedString));
Solution 3
You can also use this code:
String actualString = driver.findElement(By.xpath("xpath")).getText();
Assert.assertTrue(actualString.contains("specific text"));
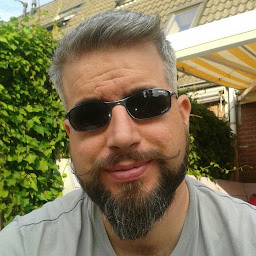
Hugo
Updated on July 05, 2022Comments
-
Hugo almost 2 years
I have a page that I know contains a certain text at a certain xpath. In firefox I use the following code to assert that the text is present:
assertEquals("specific text", driver.findElement(By.xpath("xpath)).getText());
I'm asserting step 2 in a form and confirming that a certain attachment has been added to the form. However, when I use the same code in Chrome the displayed output is different but does contain the specific text. I get the following error:
org.junit.ComparisonFailure: expected:<[]specific text> but was:<[C:\fakepath\]specific text>
Instead of asserting something is true (exactly what I'm looking for) I'd like to write something like:
assert**Contains**("specific text", driver.findElement(By.xpath("xpath)).getText());
The code above does not work obviously but I can't find how to get this done.
Using Eclipse, Selenium WebDriver and Java
-
stackhelper101 over 8 yearsI believe this question was answered [here](stackoverflow.com/questions/1092219/…
-
-
Hugo over 8 yearsThank you! I used the following and that worked fine: assertTrue(driver.findElement(By.xpath("xpath")).getText().contains("specific text"));
-
Andrio over 8 yearsYou can optionally add a second parameter, with a custom error message for if it fails. For example:
assertTrue(s.contains("specific text"), "String did not contain the required text.");
-
user1622681 almost 3 yearsI know this is an old post but has anyone done the same thing in C#? I would love to see that code.