How to assign a value from a C# static method to a label
Solution 1
If the method must be static for some reason, the main approach here would be to pass any required state into the method - i.e. add a parameter to the method that is either the label or (better) some typed wrapper with a settable property like .Greeting
:
public static string Greet(string name, YourType whatever)
{
string greeting = "welcome ";
whatever.Greeting = string.Concat(greeting, name);
return name;
}
(where YourType
could be your control, or could be an interface allowing re-use)
What you don't want to do is use static state or events - very easy to get memory leaks etc that way.
For example:
public static string Greet(string name, IGreetable whatever)
{
string greeting = "welcome ";
whatever.Greeting = string.Concat(greeting, name);
return name;
}
public interface IGreetable {
string Greeting {get;set;}
}
public class MyForm : Form, IGreetable {
// snip some designer code
public string Greeting {
get { return helloLabel.Text;}
set { helloLabel.Text = value;}
}
public void SayHello() {
Greet("Fred", this);
}
}
Solution 2
Either non-static:
public string Greet(string name)
{
const string greeting = "welcome ";
string concat = string.Concat(greeting, name);
Label1.Text = concat;
return name;
}
Or still static passing the label like Greet("John", Label1)
:
public static string Greet(string name, Label label)
{
const string greeting = "welcome ";
string concat = string.Concat(greeting, name);
label.Text = concat;
return name;
}
But not sure why you need to return the name in either case...if you had it when calling the function, you already have it in the scope you'd be returning to. Example:
var name = "John";
Greet(name);
//can still call name here directly
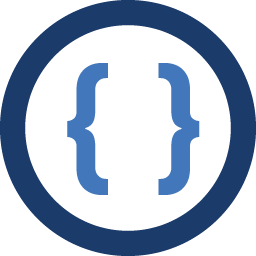
Admin
Updated on June 18, 2022Comments
-
Admin about 2 years
I have the following static function in c#
public static string Greet(string name) { string greeting = "welcome "; // is it possible to pass this value to a label outside this static method? string concat = string.Concat(greeting, name); //error Label1.text = concat; //I want to return only the name return name; }
As you can see in the comments, I want to retain only the name as the return value, however I want to be able to take out the value of the concat variable to asign it to a label, but when i try the compiler refuses, can it be done? Is there a work around?
Thank you.
-
Admin over 14 yearsthank you Nick, That is just a simplified example with just the name. The reason it is static is because it is decorated with the [WebMethod] attribute. However I don't know if there is a way to access a Page method from Ajax without declaring it as static.
-
Nick Craver over 14 years@tika - Ah, in that case, yes it has to be static.
-
Admin over 14 yearsThank you Marc, that really makes sense. I am trying it out. :)
-
Admin over 14 yearsHi Nick, I try this example but the compiler complains that the ystem.Web.UI.WebControls.Label' does not contain a definition for 'text'. I am sorry I am such a noob, I am just learning.
-
Admin over 14 yearsHi Marc, thank you. Ok I make a wrapper and I can assign the value to it: public class MyType { private string x; public string Greeting { get { return x; } set { x = value; } } } However I am still having difficulty figuring out how to assign the wrapper property to the Label right after the Greet method executes. thanks in advance.
-
Admin over 14 yearsHi Nismoto, You are right, but this is a different scenario, I wanted to be able to get information without using the return value, the return value is used already. I have tried calling Page methods in ASP.NET 3.5 with Ajax and they do not require to be static, however in .NET 2.0 they just don't work unless they are declared as static. but I guess that will be another separate question.