How to authenticate to Visual Studio Team Services with the new basic authentication from a .Net Windows Service?
First of all, you need to have at least Visual Studio 2012 Update 1 installed on your machine. It includes an updated Microsoft.TeamFoundation.Client.dll
assembly with the BasicAuthCredential
class.
Here's the code to do it, from Buck's blog post How to connect to Team Foundation Service.
using System;
using System.Net;
using Microsoft.TeamFoundation.Client;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
NetworkCredential netCred = new NetworkCredential(
"[email protected]",
"yourbasicauthpassword");
BasicAuthCredential basicCred = new BasicAuthCredential(netCred);
TfsClientCredentials tfsCred = new TfsClientCredentials(basicCred);
tfsCred.AllowInteractive = false;
TfsTeamProjectCollection tpc = new TfsTeamProjectCollection(
new Uri("https://YourAccountName.visualstudio.com/DefaultCollection"),
tfsCred);
tpc.Authenticate();
Console.WriteLine(tpc.InstanceId);
}
}
}
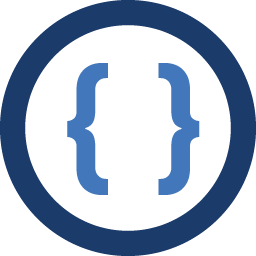
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am trying to access https://visualstudio.com (formerly known as https://tfs.visualstudio.com, http://www.tfspreview.com) from my Windows Service written on .NET.
I want to use the new basic authentication but I couldn't find a way to do it.
I found a lot of links to the blog post Team Foundation Service updates - Aug 27 but it is using the Team Explorer Everywhere Java client for TFS.
Is there a new version of the TFS .NET Object Model to support the basic authentication?
By the way I've successively logged in with the service account. This answer was very useful.
-
Admin almost 10 yearsNote that this requires an
https:
connection. -
Arno Peters over 7 yearsI've had trouble getting this to work - Apparently when you use your Microsoft Account credentials the supplied values will not work as expected. To authenticate against VSO 2015 I had to enable "alternate credentials" so that I could change the username to a non-email-address format. After that this code worked fine.
-
afr0 over 7 yearsagree with Volkirith. this doesn't work with VS2015 now need alternate credentials setup first before you can actually run it.
-
csnate almost 7 yearsThis is kind of an old thread, but if you go over SSL then try using the SimpleWebTokenCredential instead of the NetworkCredential and BasicAuthCredential.