how to automatically scroll down a html page?
Solution 1
You can use .scrollIntoView()
for this. It will bring a specific element into the viewport.
Example:
document.getElementById( 'bottom' ).scrollIntoView();
Demo: http://jsfiddle.net/ThinkingStiff/DG8yR/
Script:
function top() {
document.getElementById( 'top' ).scrollIntoView();
};
function bottom() {
document.getElementById( 'bottom' ).scrollIntoView();
window.setTimeout( function () { top(); }, 2000 );
};
bottom();
HTML:
<div id="top">top</div>
<div id="bottom">bottom</div>
CSS:
#top {
border: 1px solid black;
height: 3000px;
}
#bottom {
border: 1px solid red;
}
Solution 2
You can use two different techniques to achieve this.
The first one is with javascript: set the scrollTop property of the scrollable element (e.g. document.body.scrollTop = 1000;
).
The second is setting the link to point to a specific id in the page e.g.
<a href="mypage.html#sectionOne">section one</a>
Then if in your target page you'll have that ID the page will be scrolled automatically.
Solution 3
here is the example using Pure JavaScript
function scrollpage() {
function f()
{
window.scrollTo(0,i);
if(status==0) {
i=i+40;
if(i>=Height){ status=1; }
} else {
i=i-40;
if(i<=1){ status=0; } // if you don't want continue scroll then remove this line
}
setTimeout( f, 0.01 );
}f();
}
var Height=document.documentElement.scrollHeight;
var i=1,j=Height,status=0;
scrollpage();
</script>
<style type="text/css">
#top { border: 1px solid black; height: 20000px; }
#bottom { border: 1px solid red; }
</style>
<div id="top">top</div>
<div id="bottom">bottom</div>
Solution 4
Use document.scrollTop
to change the position of the document. Set the scrollTop
of the document
equal to the bottom
of the featured section of your site
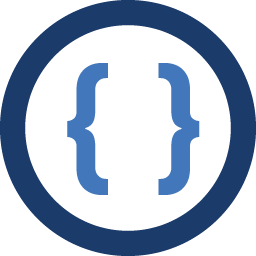
Admin
Updated on December 23, 2020Comments
-
Admin over 3 years
I'm trying to start each page after the homepage about 500px down, similar to this website: http://unionstationdenver.com/
You'll notice when viewing pages after the homepage, you're automatically scrolled down without notice but you can than scroll up to revel the featured slider again.
I've played with scrolledHeight but I dont think that is what I need????
Basically I have a featured section that is on top of all my content pages, but you shouldn't be able to see this section until you scroll up. Any help?
-
Luca over 12 yearsscrollTop is applicable just to a DOM element, not to the document
-
Wex over 12 yearsFor your first technique, how do you get it so it's down to the position of a specific element?
-
Admin over 12 yearsYes I have that about doing anchors but that is the last resort. I just can't tell how these guys did it unionstationdenver.com
-
Luca over 12 years@Wex in plain javascript you can find the position of an element inside a document like this:
var topPosition = document.getElementById("myElement").offsetTop;
-
Luca over 12 years@Hambone you can see in this script link that they are using the scrollTo method of the window object. More info: scrollTo on mozilla
-
jfritz42 over 10 yearsThis solution works for me under IE but not Chrome. Any ideas?
-
jfritz42 over 10 yearsMore info: it works in Chrome on the first page load but not on refresh. I think it's due to this bug: code.google.com/p/chromium/issues/detail?id=280460. If I hard-refresh the page it works, i.e. clicking in the URL bar and hitting enter. But soft-resets like the page refreshing or clicking the refresh button (or hitting F5) do not work.