How to both read and write a file in C#
Solution 1
You need a single stream, opened for both reading and writing.
FileStream fileStream = new FileStream(
@"c:\words.txt", FileMode.OpenOrCreate,
FileAccess.ReadWrite, FileShare.None);
Solution 2
var fs = File.Open("file.name", FileMode.OpenOrCreate, FileAccess.ReadWrite);
var sw = new StreamWriter(fs);
var sr = new StreamReader(fs);
...
fs.Close();
//or sw.Close();
The key thing is to open the file with the FileAccess.ReadWrite flag. You can then create whatever Stream/String/Binary Reader/Writers you need using the initial FileStream.
Solution 3
This thread seems to answer your question : simultaneous-read-write-a-file
Basically, what you need is to declare two FileStream, one for read operations, the other for write operations. Writer Filestream needs to open your file in 'Append' mode.
Solution 4
you can try this:"Filename.txt" file will be created automatically in the bin->debug folder everytime you run this code or you can specify path of the file like: @"C:/...". you can check ëxistance of "Hello" by going to the bin -->debug folder
P.S dont forget to add Console.Readline() after this code snippet else console will not appear.
TextWriter tw = new StreamWriter("filename.txt");
String text = "Hello";
tw.WriteLine(text);
tw.Close();
TextReader tr = new StreamReader("filename.txt");
Console.WriteLine(tr.ReadLine());
tr.Close();
Solution 5
Made an improvement code by @Ipsita - for use asynchronous read\write file I/O
readonly string logPath = @"FilePath";
...
public async Task WriteToLogAsync(string dataToWrite)
{
TextReader tr = new StreamReader(logPath);
string data = await tr.ReadLineAsync();
tr.Close();
TextWriter tw = new StreamWriter(logPath);
await tw.WriteLineAsync(data + dataToWrite);
tw.Close();
}
...
await WriteToLogAsync("Write this to file");
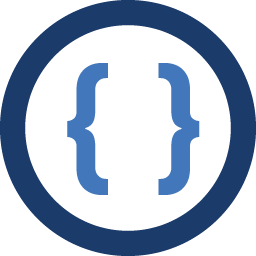
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I want to both read from and write to a file. This doesn't work.
static void Main(string[] args) { StreamReader sr = new StreamReader(@"C:\words.txt"); StreamWriter sw = new StreamWriter(@"C:\words.txt"); }
How can I both read from and write to a file in C#?
-
ScottS about 15 yearsFileShare.ReadWrite is not neccesary, and is likely undeseriable, it will allow other applications to Read and Write your file while you are using it. Generally FileShare.None is preferred when writing to a file, to prevent others from accessing a file while you work on it.
-
Samuel about 15 years@ScottS: I agree as far as the ReadWrite is necessary as you can let the constructor figure out the sharing mode.
-
Yehonatan over 10 yearsThank you both! you really helped me!