How to break to new line in Fortran when printing?
Solution 1
There are several ways to print two lines of output.
program foo
print *, 'This is the first line'
print *, 'This is the second line'
end program
is one way to achieve what you want. Another is to do
program foo
write(*,'(A,/,A)') 'This is the first line', 'This is the second line'
end program foo
And, yet another way
program foo
write(*,'(A)') 'A' // achar(13) // achar(10) // 'B'
end program foo
And with some compilers you can use options
program foo
write(*,'(A)') 'A\r\nB'
end program foo
Compiling with the following options yields:
$ gfortran -o z -fbackslash a.f90 && ./z
A
B
Solution 2
There are a number of ways to manage what you want. We can either print blank records or explicitly add a newline character.
A newline character is returned by the intrinsic function NEW_LINE
:
print '(2A)', 'First line', NEW_LINE('a')
print '(A)', 'Second line'
NEW_LINE('a')
is likely to have an effect like ACHAR(10)
or CHAR(10,KIND('a'))
.
A blank record can be printed by having no output item:
print '(A)', 'First line'
print '(A)'
print '(A)', 'Second line'
Or we can use slash editing:
print '(A,/)', 'First line'
print '(A)', 'Second line'
If we aren't using multiple print statements we can even combine the writing using these same ideas. Such as:
print '(A,:/)', 'First line', 'Second line'
print '(*(A))', 'First line', NEW_LINE('a'), NEW_LINE('a'), 'Second line'
NEW_LINE('a')
could also be used in the format string but this doesn't seem to add much value beyond slash editing.
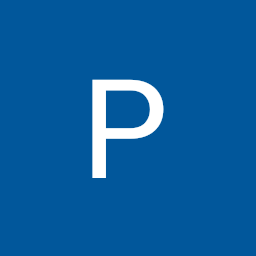
Pro Academy
Updated on June 11, 2022Comments
-
Pro Academy 12 months
It is very simple query but I'm not able to find the exact solution. How to break to new line when printing in Fortran?
for example
print*,'This is first line' print*,'This is second line'
I want following output
This is first line This is Second line
That is add space between two lines.
In java we use
\n
and in html using<br>
does the job..but how to achieve same in Fortran?-
Ross over 3 yearsPlease try to be more clear in your question - formatting can help (stackoverflow.com/editing-help)
-
-
Pro Academy over 3 yearsNO ....I want the space or line between two print statement...? is there any way to achieve this...in html i can use <br> but in fortran I didn't see any option instead if I write print statement separately at some distance below.
-
evets over 3 yearsOf course, just add
print *
between the print statements. BTW, your question wasn't stated too clearly. :( -
evets over 3 yearsAh, good catch with
NEW_LINE
. I had forgotten about that intrinsic subprogram.