How to build a custom Email action handler in Flutter for Firebase Authentication
To complete the email action, you need to fetch the oobCode
from URL parameters and use applyActionCode
method (checkActionCode
can be used before applyActionCode
to make sure the oobCode is valid, usually not required as applyActionCode
will throw an error on getting an invalid code as well):
var actionCode = "" // Get from URL
try {
await auth.checkActionCode(actionCode);
await auth.applyActionCode(actionCode);
// If successful, reload the user:
auth.currentUser.reload();
} on FirebaseAuthException catch (e) {
if (e.code == 'invalid-action-code') {
print('The code is invalid.');
}
}
If you are using dynamic links, you can get it this way:
//Get actionCode from the dynamicLink
final Uri deepLink = dynamicLink?.link;
var actionCode = deepLink.queryParameters['oobCode'];
You can refer the documentation for more information.
Alternatively, you can also use the Firebase REST API to verify the email links if you need to verify the oobCode from a Cloud function or server.
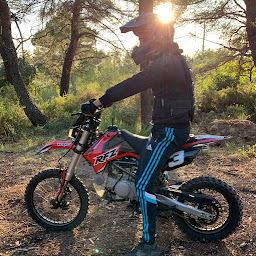
Tom3652
Updated on December 17, 2022Comments
-
Tom3652 over 1 year
I am very new to web development and i am building a website with
Flutter
.
I am aware of theRouting
/Navigator
system.
I would like to know how can i create such page inFlutter
to handle Firebase email actions :https://example.com/usermgmt?mode=resetPassword&oobCode=ABC123&apiKey=AIzaSy...&lang=fr
I am following this documentation.
It is stated here that:
Firebase adds several query parameters to your action handler URL when it generates user management emails.
However i don't understand if it's up to me to build the full
url
in myFlutter
Routes
or simply provide the endpoint/usermgmt
and Firebase is automatically adding the params ?I have tried to build a page in my website that addresses :
https://example.com/usermgmt
and it's working, the page exists hosted in my website.
Now if i add the Firebase params, it returns a404 not found
.What should i do ?
EDIT : I have made the UI working, it doesn't return a
404 not found
anymore.
I have done this for those interrested :onGenerateRoute: (settings) { Widget? authPage; if (settings.name != null) { var uriData = Uri.parse(settings.name!); //uriData.path will be the path and uriData.queryParameters will hold query-params values print("Param in query : " + uriData.queryParameters.toString()); switch (uriData.path) { case '/auth': authPage = AuthHandlerPage(); break; } } if (authPage != null) { return MaterialPageRoute( builder: (BuildContext context) => authPage!); } },
I simply need to handle the links according to the params now.