How to call rails method from view on button or link click
The view and the controller are independent of each other. In order to make a link execute a function call within the controller, you need to do an ajax call to an endpoint in the application. The route should call the ruby method and return a response to the ajax call's callback, which you can then interpret the response.
As an example, in your view:
<%= link_to 'Add', '#', :onclick => 'sum_fn()' %>
<%= javascript_tag do %>
function sum_fn() {
/* Assuming you have jQuery */
$.post('/ajax/sum.json', {
num1: 100,
num2: 50
}, function(data) {
var output = data.result;
/* output should be 150 if successful */
});
}
<% end %>
In your routes.rb
add a POST
route for your ajax call endpoint.
post '/ajax/sum' => 'MyController#ajax_sum'
Then suppose you have a MyController class in mycontroller.rb
. Then define the method ajax_sum
.
class MyController < ApplicationController
# POST /ajax/sum
def ajax_sum
num1 = params["num1"].to_i
num2 = params["num2"].to_i
# Do something with input parameter and respond as JSON with the output
result = num1 + num2
respond_to do |format|
format.json {render :json => {:result => result}}
end
end
end
Hope that hopes!
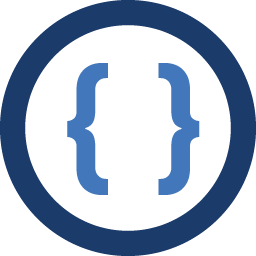
Admin
Updated on June 18, 2022Comments
-
Admin almost 2 years
Basically, I'm trying to have a rails method execute when the user clicks on a link (or a button or some type of interactive element).
I tried putting this in the view:
<%= link_to_function 'Add' , function()%>
But that didn't seem to work. It ended up simply calling the function without a user even clicking on the "Add" link.
I tried it with link_to as well, but that didn't work either. I'm starting to think there isn't a clean way to do this. Anyway, thanks for your help.
PS. I defined the method in the ApplicationController and it is a helper method.
-
sealocal over 9 yearsI think you have to use parens to call the function:
:onclick => 'sum_fn()'
. Right? -
Bobby over 9 yearsFixed thanks! Using
onclick
is probably not the best way of registering a click handler. It should instead be registered with Javascript, but for the purposes of demonstration, I think this is enough. -
Justin Harris about 9 yearsThanks but you're missing a closing bracket on the post function.