How to change BottomNavigationBar background colour?
6,662
Solution 1
try this
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatefulWidget {
State<StatefulWidget> createState(){
return MyAppState();
}
}
class MyAppState extends State<MyApp>{
int _selectedPage = 0;
final _pageOptions = [
Text('Item1'),
Text('Item2'),
Text('Item3')
];
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'sddsd',
theme: ThemeData(
primaryColor: Colors.blueAccent,
fontFamily: "Google Sans"
),
home: Scaffold(
appBar: AppBar(
title:Text("LQ2018"),
//backgroundColor: Colors.blueAccent,
),
body: _pageOptions[_selectedPage],
bottomNavigationBar: BottomNavigationBar(
//fixedColor: Colors.blueAccent,
type: BottomNavigationBarType.shifting,
currentIndex: _selectedPage,
onTap: (int index){
setState(() {
_selectedPage= index;
});
},
items: [
BottomNavigationBarItem(icon: Icon(Icons.supervised_user_circle), title: Text('Players'),backgroundColor: Colors.blueAccent),
BottomNavigationBarItem(icon: Icon(Icons.whatshot), title: Text('Trending'),backgroundColor: Colors.blueAccent),
BottomNavigationBarItem(icon: Icon(Icons.access_time), title: Text('Highlights'),backgroundColor: Colors.blueAccent)
]
),
),
);
}
}
Solution 2
Wrap your BottomNavigationBar in a Theme and set the canvasColor in data of the theme.
@override
Widget build(BuildContext context) {
return Scaffold(
bottomNavigationBar: Theme(
data: Theme.of(context).copyWith(
canvasColor: Colors.blue,
textTheme: Theme.of(context)
.textTheme
.copyWith(caption: TextStyle(color: Colors.black54))),
child: BottomNavigationBar(
type: BottomNavigationBarType.fixed,
currentIndex: currentIndex,
fixedColor: Colors.green,
onTap: (value) {},
items: [
BottomNavigationBarItem(
icon: Icon(Icons.add),),
],
)),
);
}
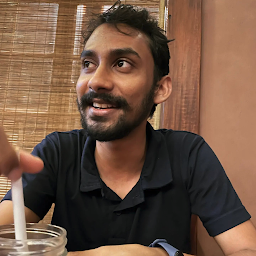
Author by
Suvin Nimnaka Sukka
Updated on December 08, 2022Comments
-
Suvin Nimnaka Sukka over 1 year
Im making a simple app with tab bars. I need to change Bottom Navigation Bar's background colour to blue. The rest of the app should be in white background and navigation bar should be blue backgrounded. How should I do that? Setting canvasColor in ThemeData didnt work.
Heres my code:
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatefulWidget { State<StatefulWidget> createState(){ return MyAppState(); } } class MyAppState extends State<MyApp>{ int _selectedPage = 0; final _pageOptions = [ Text('Item1'), Text('Item2'), Text('Item3') ]; @override Widget build(BuildContext context) { return MaterialApp( title: 'sddsd', theme: ThemeData( primaryColor: Colors.blueAccent, fontFamily: "Google Sans" ), home: Scaffold( appBar: AppBar( title:Text("LQ2018"), backgroundColor: Colors.blueAccent, ), body: _pageOptions[_selectedPage], bottomNavigationBar: BottomNavigationBar( fixedColor: Colors.blueAccent, currentIndex: _selectedPage, onTap: (int index){ setState(() { _selectedPage= index; }); }, items: [ BottomNavigationBarItem(icon: Icon(Icons.supervised_user_circle), title: Text('Players')), BottomNavigationBarItem(icon: Icon(Icons.whatshot), title: Text('Trending')), BottomNavigationBarItem(icon: Icon(Icons.access_time), title: Text('Highlights')) ] ), ), ); } }
-
Suvin Nimnaka Sukka over 5 yearsYes! Working perfectly fine. Thanks
-
Suvin Nimnaka Sukka over 5 yearsThanks and I found adding "type: BottomNavigationBarType.shifting" property and then setting up background colour for Tabitems is easy :)