How to change the stroke width of a shape programmatically in Android?
18,805
Solution 1
You may need to create it programmatically
ShapeDrawable circle = new ShapeDrawable( new OvalShape() );
you need to set the properties after this, ( padding, color, etc) then change its stroke
circle.getPaint().setStrokeWidth(12);
then set it as the background for the view
textview.setBackgroundDrawable(circle);
Solution 2
Do like this:
1) Get the TextView
using the usual findViewById()
:
TextView textView = (TextView) rootView.findViewById(R.id.resourceName);
2) Get the Drawable
from TextView
using getBackground()
and cast it to GradientDrawable
:
GradientDrawable backgroundGradient = (GradientDrawable) textView.getBackground();
3) Apply it a stroke using setStroke()
method (pass it the width in pixel and the color):
backgroundGradient.setStroke(5, Color.BLACK);
Whole code:
TextView textView = (TextView) rootView.findViewById(R.id.resourceName);
GradientDrawable backgroundGradient = (GradientDrawable) textView.getBackground();
backgroundGradient.setStroke(5, Color.BLACK);
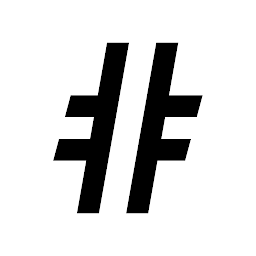
Author by
Taha Körkem
Updated on July 23, 2022Comments
-
Taha Körkem almost 2 years
This is circle.xml
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="oval"> <solid android:color="#00000000"/> <padding android:left="30dp" android:top="30dp" android:right="30dp" android:bottom="30dp" /> <stroke android:color="#439CC8" android:width="7dp" /> </shape>
This is my code:
textview.setBackgroundResource(R.drawable.circle);
I want to change the stroke thickness in my java code. How can I change it programmatically?