How to check 'late' variable is initialized in Dart
Solution 1
Unfortunately this is not possible.
From the docs:
AVOID late variables if you need to check whether they are initialized.
Dart offers no way to tell if a late variable has been initialized or assigned to. If you access it, it either immediately runs the initializer (if it has one) or throws an exception. Sometimes you have some state that’s lazily initialized where late might be a good fit, but you also need to be able to tell if the initialization has happened yet.
Although you could detect initialization by storing the state in a late variable and having a separate boolean field that tracks whether the variable has been set, that’s redundant because Dart internally maintains the initialized status of the late variable. Instead, it’s usually clearer to make the variable non-late and nullable. Then you can see if the variable has been initialized by checking for null.
Of course, if null is a valid initialized value for the variable, then it probably does make sense to have a separate boolean field.
Solution 2
Some tips I came up with from advice of different dart maintainers, and my self-analysis:
late
usage tips:
- Do not use
late
modifier on variables if you are going to check them for initialization later. - Do not use
late
modifier for public-facing variables, only for private variables (prefixed with_
). Responsibility of initialization should not be delegated to API users. EDIT: as Irhn mentioned, this rule makes sense forlate final
variables only with no initializer expression, they should not be public. Otherwise there are valid use cases for exposinglate
variables. Please see his descriptive comment! - Do make sure to initialize
late
variables in all constructors, exiting and emerging ones. - Do be cautious when initializing a
late
variable inside unreachable code scenarios. Examples:-
late
variable initialized inif
clause but there's no initialization inelse
, and vice-versa. - Some control-flow short-circuit/early-exit preventing execution to reach the line where
late
variable is initialized.
-
Please point out any errors/additions to this.
Enjoy!
Sources:
- eernstg's take
- Hixie's take
- lrhn's take
- leafpetersen's final verdict as of 2021 10 22
- Effective Dart
- Self-analysis on how to approach this with some common-sense.
Solution 3
Someone may kill you if they encounter it down the road, but you can wrap it in a try/catch/finally to do the detection. I like it better than a separate boolean.
We have an instance where a widget is disposed if it fails to load and contains a late controller that populates on load. The dispose fails as the controller is null, but this is the only case where the controller can be null. We wrapped the dispose in a try catch to handle this case.
Solution 4
You can create a Late class and use extensions like below:
import 'dart:async';
import 'package:flutter/foundation.dart';
class Late<T> {
ValueNotifier<bool> _initialization = ValueNotifier(false);
late T _val;
Late([T? value]) {
if (value != null) {
this.val = value;
}
}
get isInitialized {
return _initialization.value;
}
T get val => _val;
set val(T val) => this
.._initialization.value = true
.._val = val;
}
extension LateExtension<T> on T {
Late<T> get late => Late<T>();
}
extension ExtLate on Late {
Future<bool> get wait {
Completer<bool> completer = Completer();
this._initialization.addListener(() async {
completer.complete(this._initialization.value);
});
return completer.future;
}
}
Create late variables with isInitialized property:
var lateString = "".late;
var lateInt = 0.late;
//or
Late<String> typedLateString = Late();
Late<int> typedLateInt = Late();
and use like this:
print(lateString.isInitialized)
print(lateString.val)
lateString.val = "initializing here";
Even you can wait for initialization with this class:
Late<String> lateVariable = Late();
lateTest() async {
if(!lateVariable.isInitialized) {
await lateVariable.wait;
}
//use lateVariable here, after initialization.
}
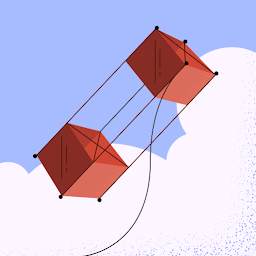
towhid
Love to design and develop tools that help others to solve their problems.
Updated on June 06, 2022Comments
-
towhid almost 2 years
In kotlin we can check if the 'late' type variables are initialized like below
lateinit var file: File if (this::file.isInitialized) { ... }
Is it possible to do something similar to this in Dart..?
-
Christopher Moore about 3 yearsI think you should emphasize the solution shown in this quote. I would recommend adding at the end or bolding to use a non-late and nullable variable.
-
Mabsten almost 3 yearsFor the discussion by the Dart team on the choice not to allow (at least for now) to check if a
late
variable has been initialized, see the interesting issue #324 - Should we provide a way to query the status of late variables? -
emanuel sanga about 2 yearsto make it appear cleaner, I have created a separate method for it.... Methods appear beautiful
-
Lalit Fauzdar almost 2 yearsOh what a bloody mess, Can't declare variables getting data from internet as
late final
and filtering makes it a mess of original data loss.