How to check bluetooth connection state with paired device in Android
Solution 1
I faced the same problem as I am working on an app which may use TTS while it is running. I think there is no way to check if there is any bluetooth device connected by the BluetoothAdapter class immediately except creating a broadcast receiver and monitor the changes of status of bluetooth.
After scratching my head for a few hours, I found a quite subtle way to solve this problem. I tried, it works pretty well for me.
AudioManager audioManager = (AudioManager) getApplicationContext.getSystemService(Context.AUDIO_SERVICE);
if (audioManager.isBluetoothA2dpOn()) {
//audio is currently being routed to bluetooth -> bluetooth is connected
}
Source: http://developer.android.com/training/managing-audio/audio-output.html
Solution 2
I think it's to late for answer to your question but I think can helps somebody :
If you use Thread you have to create a BroadcastReceiver in your main activity on create :
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_digital_metrix_connexion);
BroadcastReceiver bState = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if(action.equals(BluetoothAdapter.ACTION_CONNECTION_STATE_CHANGED))
{
int state = intent.getIntExtra(BluetoothAdapter.EXTRA_STATE,
BluetoothAdapter.ERROR);
switch (state)
{
case BluetoothAdapter.ACTION_ACL_CONNECTED:
{
//Do something you need here
System.out.println("Connected");
break;
}
default:
System.out.println("Default");
break;
}
}
}
};
}
BluetoothAdapter.STATE_CONNECTED
is one state over many, for exemple it's possible to check if device connecting or disconnecting thanks to BluetoothAdapter.ACTION_ACL_DISCONNECTED
or BluetoothAdapter.ACTION_ACL_DISCONNECTED_REQUEST
.
After, you have to create a filter in your thread class or in you main activity if you don't use thread :
IntentFilter filter = new IntentFilter(BluetoothAdapter.ACTION_STATE_CHANGED);
This filter check the bluetoothadapter state.
And now you have to register your filter so if you use thread pass context in parameter of your thread like this context.registerReceiver(bState,filter);
or in your main Activity : registerReceiver(bState,filter);
If you have any question don't hesitate to ask me.
Hope I helps you somebody.
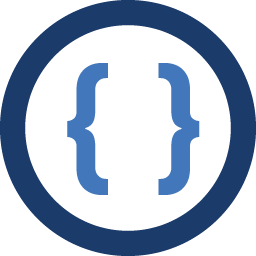
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I develop an bluetooth app which will connect to a paired device and send a message, but I have to test connection before. I've tried many options, but nothing works in good way. So could you send me any example of code which can do it? I made an thread, but I can't get an good state of connection to build an "if" function. Here is the code:
package com.example.szukacz; import java.lang.reflect.Method; import java.util.Set; import android.app.Activity; import android.bluetooth.BluetoothAdapter; import android.bluetooth.BluetoothDevice; import android.bluetooth.BluetoothSocket; import android.content.BroadcastReceiver; import android.content.Context; import android.content.Intent; import android.os.Bundle; import android.os.Handler; import android.os.Message; import android.util.Log; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.LinearLayout; import android.widget.TextView; import android.widget.Toast; public class MainActivity extends Activity { LinearLayout sparowaneUrzadzenia; public void lokalizowanie() { Intent intencja = new Intent(this, Lokalizator.class); startActivity(intencja); } public void parowanie(View v) { Intent intencja = new Intent(this, Parowanie.class); startActivity(intencja); } boolean isRunning; Handler handler = new Handler() { @Override public void handleMessage(Message msg) { String status = (String)msg.obj; if(status == "polaczony") { alarm(); showToast("prawda, zwraca" + status); } else { showToast("wykonanie x, zwraca: " + status); }; } }; public void alarm() { showToast("Alarm!!!"); } @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); sparowaneUrzadzenia = (LinearLayout) findViewById(R.id.listaUrzadzenGlowna); pokazSparowane(); } public void onStop() { super.onStop(); isRunning = false; } public void onStart() { super.onStart(); Thread testPolaczen = new Thread(new Runnable() { public void run() { try { for(int i = 0; i < 1000000; i++) { Thread.sleep(5000); testujPolaczenia(); int stan = 0; String status = Integer.toString(stan); Message msg = handler.obtainMessage(1, (String)status); if(isRunning == true) { handler.sendMessage(msg); } } } catch (Throwable t) { // watek stop } } }); isRunning = true; testPolaczen.start(); } private void testujPolaczenia() { } public void pokazSparowane(){ /* * Wyświetlanie listy sparowanych urządzeń . * */ Log.d("INFO","Sparowane dla tego urzÄ…dzenia"); BluetoothAdapter mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter(); Set<BluetoothDevice> pairedDevices = mBluetoothAdapter.getBondedDevices(); if (pairedDevices.size() > 0) { for (BluetoothDevice device : pairedDevices) { Log.d("INFO",device.getName()+" - "+device.getAddress()); // dodawanie urzadzen do listy Button urzadzenie = new Button(getApplicationContext()); urzadzenie.setText(device.getName()); // urzadzenie.setTextColor(0xffffff); //jak ustawic na czarny kolor napsisów ? urzadzenie.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { showToast("klik"); lokalizowanie(); } }); sparowaneUrzadzenia.addView(urzadzenie); } } else { showToast("brak sparowanych urzadzen"); } } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu); return true; } private void showToast(String message) { Toast.makeText(getApplicationContext(), message, Toast.LENGTH_SHORT).show(); } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } }
thanks!