How to check for html5 video support?
Solution 1
use:
<script>
alert(!!document.createElement('video').canPlayType);
</script>
if it alerts true implies that your browser supports HTML5 video tag
Here is the url to check HTML5 Browser compatibility http://www.html5test.com/ Open the url in your browser to test how well your browser supports html5
Solution 2
Just a little refinement of sweets-BlingBling's answer : sorry - I can't comment yet :(
var isHTML5Video = (typeof(document.createElement('video').canPlayType) != 'undefined') ? true : false;
or even simpler (thanks digitalBath - as always I can't see the wood for the trees :) )
var isHTML5Video = (typeof(document.createElement('video').canPlayType) != 'undefined');
Solution 3
Have a look at Modernizer: http://www.modernizr.com/
There, you get APIs as easy as
if (Modernizr.video) {
// html5 video available
}
But many more features and more suitable APIs as well.
Solution 4
One way is to embed the html5 tags, and then put the alternate video viewer within the video tags as a "fallback". The fallback will get displayed if a browser doesnt recognize the tag. Its not strictly 'detecting' html5 video support, but may suit your needs.
<video src='...'>
<embed flash player instead>
</video>
Solution 5
I use a slight variation of @sweets-BlingBling's answer, which is:
var hasVideo = !!document.createElement('video').canPlayType &&
!!document.createElement('video').canPlayType('video/mp4')
This also checks whether the media type 'video/mp4'
is actually playable (change this if your video has another media type, like 'video/webm'
or 'video/ogg'
). The method returns an empty String if the video definitely cannot be played and 'probably'
or 'maybe'
(actually, both results mean yes
in most instances) otherwise. I had to add this for Chrome 41 (seemingly used in google crawler), which has canPlayType
, but cannot play mp4
videos.
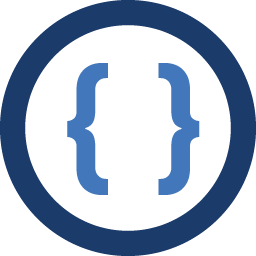
Admin
Updated on July 16, 2022Comments
-
Admin almost 2 years
Is there any JavaScript or any other way of checking for html5 video support?
-
digitalbath over 10 yearsFor what it's worth, "{boolean_expression} ? true : false" is no different than "{boolean_expression}"
-
D. Dan over 5 yearsIE11 returns true, but doesn't play hls stream/video with videojs. Having a flash fallback is not an option. Any ideas?
-
Randy over 5 yearsJust curious if this line of code will generate java script errors when debugging a page, IF the browser actually was not capable of parsing "document.createElement('video').canPlayType", because it was too old for HTML5. I guess it would always generate "false" for the variable, but would it choke in doing so?