How to check if a string is a palindrome?
Solution 1
Just reverse the string and compare it against the original
string_to_check = input("Enter a string")
if string_to_check == string_to_check[::-1]:
print("This is a palindrome")
else:
print("This is not a palindrome")
Solution 2
I had to make a few changes in your code to replicate the output you said you saw.
Ultimately, what you want is that the message be displayed only at the end of all the comparisons. In your case, you had it inside the loop, so each time the loop ran and it hit the if
condition, the status message was printed. Instead, I have changed it so that it only prints when the two pointers index
and p
are at the middle of the word.
str = input("Enter the string: ")
l = len(str)
p = l-1
index = 0
while index < p:
if str[index] == str[p]:
index = index + 1
p = p-1
if index == p or index + 1 == p:
print("String is a palindrome")
else:
print("string is not a palindrome")
break
Solution 3
I see that most of the solutions in the internet is where either the string is taken as an input or the string is just reversed and then tested. Below is a solution that considers two points: 1) The input is a very large string therefore, it cannot be just taken from a user. 2) The input string will have capital casing and special characters. 3) I have not looked into the complexity and see further improvement. Would invite suggestions.
def isPalimdromeStr(self, strInput):
strLen = len(strInput)
endCounter = strLen - 1
startCounter = 0
while True:
# print(startCounter, endCounter)
# print(strInput[startCounter].lower(), strInput[endCounter].lower())
while not blnValidCh(self, strInput[startCounter].lower()):
startCounter = startCounter + 1
while not blnValidCh(self, strInput[endCounter].lower()):
endCounter = endCounter - 1
# print(startCounter, endCounter)
# print(strInput[startCounter].lower() , strInput[endCounter].lower())
if (strInput[startCounter].lower() != strInput[endCounter].lower()):
return False
else:
startCounter = startCounter + 1
endCounter = endCounter - 1
if (startCounter == strLen - 1):
return True
# print("---")
def blnValidCh(self, ch):
if rePattern.match(ch):
return True
return False
global rePattern
rePattern = re.compile('[a-z]')
blnValidPalindrome = classInstance.isPalimdromeStr("Ma##!laYal!! #am")
print("***")
print(blnValidPalindrome)
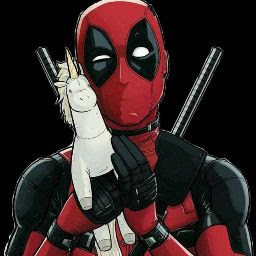
Kawin M
By day: Biology major By night: Loves coding By midnight: Loves sleeping
Updated on June 15, 2022Comments
-
Kawin M almost 2 years
I have a code to check whether a word is palindrome or not:
str = input("Enter the string") l = len(str) p = l-1 index = 0 while index < p: if str[index] == str[p]: index = index + 1 p = p-1 print("String is a palindrome") break else: print("string is not a palindrome")
If a word is inputted, for example : rotor , I want the program to check whether this word is palindrome and give output as "The given word is a palindrome".
But I'm facing problem that, the program checks first r and r and prints "The given word is a palindrome" and then checks o and o and prints "The given word is a palindrome". It prints the result as many times as it is checking the word.
I want the result to be delivered only once. How to change the code?