How to check if an attribute exists in a XML file using XSL
Solution 1
Here is a very simple way to do conditional processing using the full power of XSLT pattern matching and exclusively "push" style, and this even avoids the need to use conditional instructions such as <xsl:if>
or <xsl:choose>
:
<xsl:stylesheet version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:output omit-xml-declaration="yes" indent="yes"/>
<xsl:template match="/root/diagram[graph[1]/@color]">
Graph[1] has color
</xsl:template>
<xsl:template match="/root/diagram[not(graph[1]/@color)]">
Graph[1] has not color
</xsl:template>
</xsl:stylesheet>
when this transformation is applied on the following XML document:
<root>
<diagram>
<graph color= "#ff00ff">
<xaxis>1 2 3 12 312 3123 1231 23 </xaxis>
<yaxis>1 2 3 12 312 3123 1231 23 </yaxis>
</graph>
<graph>
<xaxis>101 102 103 1012 10312 103123 101231 1023 </xaxis>
<yaxis>101 102 103 1012 10312 103123 101231 1023 </yaxis>
</graph>
</diagram>
</root>
the wanted, correct result is produced:
Graph[1] has color
when the same transformation is applied on this XML document:
<root>
<diagram>
<graph>
<xaxis>101 102 103 1012 10312 103123 101231 1023 </xaxis>
<yaxis>101 102 103 1012 10312 103123 101231 1023 </yaxis>
</graph>
<graph color= "#ff00ff">
<xaxis>1 2 3 12 312 3123 1231 23 </xaxis>
<yaxis>1 2 3 12 312 3123 1231 23 </yaxis>
</graph>
</diagram>
</root>
again the wanted and correct result is produced:
Graph[1] has not color
One can customize this solution and put whatever code is necessary inside the first template and if necessary, inside the second template.
Solution 2
Customize the template in one match like this
<xsl:template match="diagram/graph">
<xsl:choose>
<xsl:when test="@color">
Do the Task
</xsl:when>
<xsl:otherwise>
Do the Task
</xsl:otherwise>
</xsl:choose>
</xsl:template>**
Solution 3
<xsl:when test="graph[1]/@color">
//some processing here using graph[1]/@color values
</xsl:when>
I'm going to make a guess here since your question is missing a lot of important information, such as the context in which thie <xsl:when...
appears. If your comment is correct, what you want to do is process graph[1]/xaxis
and .../yaxis
, not graph[1]/@color
values.
Solution 4
I don't get it - apart from some slight syntax tweak towards using apply-templates:
<xsl:template match="graph[1][@color]">
<!-- your processing here -->
</xsl:template>
There's not much we can tell you without knowing what you actually want to do.
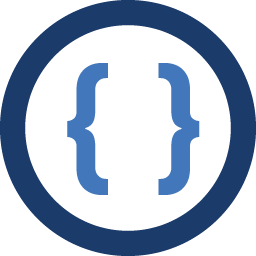
Admin
Updated on February 05, 2020Comments
-
Admin over 4 years
At runtime I can have two formats of a XML file:
<root> <diagram> <graph color= "#ff00ff"> <xaxis>1 2 3 12 312 3123 1231 23 </xaxis> <yaxis>1 2 3 12 312 3123 1231 23 </yaxis> </graph> </diagram> </root>
<root> <diagram> <graph> <xaxis>1 2 3 12 312 3123 1231 23 </xaxis> <yaxis>1 2 3 12 312 3123 1231 23 </yaxis> </graph> </diagram> </root>
Depending on the presence of the color attribute i have to process the values of the xaxis and yaxis.
I need to do this using XSL. Can anyone help me in hinting me a snippet where i can check these condtions.
I tried using
<xsl: when test="graph[1]/@color"> //some processing here using graph[1]/@color values </xsl:when>
i got an error ...