How to check if an element exists on the page in Playwright.js
Solution 1
You have two main options:
const deletes = await page.$$("text='Delete'");
if (deletes) {
// ...
}
or
const deletes = await page.$$("text='Delete'");
if (deletes.length) {
// ...
}
I'd personally leave the $$
command outside the if statement for readability, but that might me only me.
It also seems you have only one element with attribute text with value "Delete" on the page since you're not doing anything with the array that $$
returns. If so, you might use $
:
const del = await page.$("text='Delete'");
if (del) {
// ...
}
Solution 2
await expect(page.locator(".MyClass")).toHaveCount(0)
Solution 3
try {
await page.waitForSelector(selector, { timeout: 5000 })
// ...`enter code here`
} catch (error) {`enter code here`
console.log("The element didn't appear.")
}
from - https://github.com/puppeteer/puppeteer/issues/1149#issuecomment-434302298
Solution 4
await page.isVisible("text='Delete'")
maybe this is the one you're looking for.
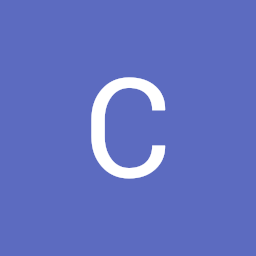
cole
Updated on April 15, 2022Comments
-
cole about 2 years
I am using playwright.js to write a script for https://target.com, and on the page where you submit shipping information, it will provide the option to use a saved address if you have gone through the checkout process previously on that target account.
I would like to enter fresh shipping information every time I run the script, so I must have playwright click delete if it exists on the page, and then enter the shipping information.
The function that is shown below works to click delete, but then it times out at
if (await page.$$("text='Delete'") != [])
rather than executing theelse
part of the function.How can I rewrite this function so that it simply checks if the element (selector:
text='Delete'
) exists, and clicks it if it does, and executes the filling part of the function if it doesn't?async function deliveryAddress() { if (await page.$$("text='Delete'") != []) { await page.click("text='Delete'", {force:true}) await deliveryAddress() } else { await page.focus('input#full_name') await page.type('input#full_name', fullName, {delay: delayms}); await page.focus('input#address_line1') await page.type('input#address_line1', address, {delay: delayms}); await page.focus('input#zip_code') await page.type('input#zip_code', zipCode, {delay: delayms}); await page.focus('input#mobile') await page.type('input#mobile', phoneNumber, {delay: delayms}); await page.click("text='Save & continue'", {force:true}) } }
-
pavel.feldman over 3 yearsYou can't compare arrays like this in JavaScript, you can check array length or use
if (await page.$('text=Delete')) {}
-
-
Admin about 2 yearsYour answer could be improved with additional supporting information. Please edit to add further details, such as citations or documentation, so that others can confirm that your answer is correct. You can find more information on how to write good answers in the help center.
-
AlexD about 2 yearsNotice that the question is "how to check if an element exists", doesn't mean to actually verify that an element is present... and this is the case. This answer will cause an exception and I am sure that's not the goal of the question. A lot of times, there is only a need to see if something is there or not, and then decide what to do based on the result of the check... and this is the reason for the question.
-
gilad 1 almost 2 yearswas the correct answerer for me. thanks. playwright.dev/docs/api/class-page#page-is-visible