How to check if data is inserted in Room Database
Solution 1
The problem was in the thread. Room doesn't allow you to run database queries in main thread. The call to insert method was inside of a try-catch block, and I was ignoring the exception. I fixed it, and here's how it reads right now.
doAsync {
val addedID = appContext.db.rallyDAO().addVehicleListItem(vehicle)
Logger.d("vehicle_lsit_item","Inserted ID $addedID")
}
Also, I reviewed the documentation, the insert method may return a Long (or List of Long in case of List passed to insert). I also changed the signature of insert, and here is the modified code
@Insert(onConflict = OnConflictStrategy.REPLACE)
fun addVehicleListItem(vehicleListItem:VehicleListItem):Long
P.S. I am using anko for doAsync
Solution 2
You can use Stetho to see your DB and Shared pref file in chrome dev tool
http://facebook.github.io/stetho/
EDIT: AS has a built-in DB tool now
Solution 3
An option you may consider, if you have not access permission issues, is to navigate directly into your sqlite instance of the device and query the tables directly there.
If you use the emulator intehgrated with Android Studio or a rooted phone you can do the following:
adb root
adb remount
cd data/data/path/of/your/application/database
sqlite3 mydb.db
Then you can query your tables
Solution 4
There are two cases regarding insertion.
Dao methods annotated with @Insert returns:
-
In case the input is only one number it returns a Long, check whether this Long equals One, if true then the Item was added successfully.
@Insert suspend fun insert( student: Student): Long
-
In case the input is varargs it returns a LongArray, check whether the size of varargs equals the LongArray size, if true then items were added successfully
@Insert suspend fun insert(vararg students: Student): LongArray
Solution 5
There are multiple ways you can test that.
- As @Ege Kuzubasioglu mentioned you can use stetho to check manually (Need minor change to code).
Pull database file from "data/data/yourpackage/databases/yourdatabase.db" to your local machine and use any applications to read the content inside the database. I personally use https://sqlitebrowser.org/. Pulling database file can be done either using the shell commands or use "Device File Explorer" from android studio.
-
Write TestCase to see if it is working. Here is an example of test case from one of my projects.
// Code from my DAO class
@Insert(onConflict = OnConflictStrategy.REPLACE) public abstract Long[] insertPurchaseHistory(List MusicOrders);
//My Test Case @Test public void insertPurchaseHistoryTest() {
// Read test data from "api-responses/music-purchase-history-response.json"
InputStream inputStream = getClass().getClassLoader()
.getResourceAsStream("api-responses/music-purchase-history-response.json");
// Write utility method to convert your stream to a string.
String testData = FileManager.readFileFromStream(inputStream);
// Convert test data to "musicOrdersResponse"
MusicOrdersResponse musicOrdersResponse = new Gson().fromJson(testData,MusicOrdersResponse.class);
// Insert inmateMusicOrders and get the list of
Long[] rowsInserted = tracksDao.insertPurchaseHistory(musicOrdersResponse.getmusicOrders());
assertThat(rowsInserted.length,Matchers.greaterThan(0));
}
Related videos on Youtube
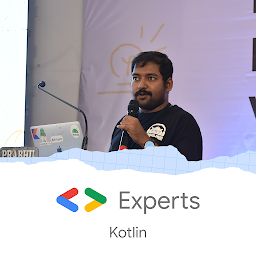
Rivu Chakraborty
Android Developer, Tech Speaker, Auther, Blogger, Community Organiser. More details: rivu.dev
Updated on July 09, 2022Comments
-
Rivu Chakraborty almost 2 years
I am using Room Database from the new Architecture components in my project. I am adding some data through dao, but when trying to retrieve it I am not getting it. Can you please suggest me how to check whether the insert was successful or not? Below are the codes to help you understand the problem.
adding to database, I checked with debugger, this statement executed successfully.
appContext.db.rallyDAO().addVehicleListItem(vehicle)
Getting null from database on this statement after insert.
val v = appContext.db.rallyDAO().getVehicleListItem(it.vehicleID)
RoomDatabase
@Database(entities = arrayOf(Rally::class, Route::class, CheckPoints::class, Vehicles::class, VehicleListItem::class), version = 1) abstract class TSDRoom: RoomDatabase() { public abstract fun rallyDAO():RallyDAO }
Inside DAO
@Insert(onConflict = OnConflictStrategy.REPLACE) fun addVehicleListItem(vehicleListItem:VehicleListItem) @Query("select * from vehicles_submitted where vehicle_id LIKE :vehicleID") fun getVehicleListItem(vehicleID:String):VehicleListItem
VehicleListItem Entity
@Entity(tableName = "vehicles_submitted", foreignKeys = arrayOf(ForeignKey(entity = Rally::class, parentColumns = arrayOf("rally_id"), childColumns = arrayOf("rally_id")))) class VehicleListItem { @PrimaryKey @ColumnInfo(name = "vehicle_id") @SerializedName("vehicle_id") var vehicleID : String = "" @ColumnInfo(name = "driver_id") @SerializedName("driver_id") var driverID : String = "" @ColumnInfo(name = "vehicle_name") @SerializedName("vehicle_name") var vehicleName : String = "" @ColumnInfo(name = "driver_name") @SerializedName("driver_name") var driverName : String = "" @ColumnInfo(name = "driver_email") @SerializedName("driver_email") var driverEmail : String = "" @ColumnInfo(name = "rally_id") @SerializedName("rally_id") var rallyID: String = "" @ColumnInfo(name = "is_passed") @SerializedName("is_passed") var isPassed = false @ColumnInfo(name = "passing_time") @SerializedName("passing_time") var passingTime:String="" }
-
zulkarnain shah almost 6 yearsIsn't there a callback for Room database operations?
-
AdamHurwitz over 5 years#2 does not work for me even though I can query by DB and clearly see there is data returned. SQLiteBrowser shows the db downloaded as empty.
-
mrts over 4 yearsMany people have upvoted and many people downvoted, so it seems to work for some and not work for others. A request for help to downvoters - can you explain what errors to you see or why you dislike this approach?
-
Mario Codes about 3 yearsI get a lot of errors on
remount
, which may all be sumarized asERROR: * Read-only file system
-
Jaimin Modi over 2 yearsWhat about insertAll ?
-
هيثم over 2 yearsThe second block inserts multiple items, it accepts a varag.
-
CodePlay about 2 yearspls note that for case 1, the return value is not always One, it is the new rowId of the inserted item.