How to check if it a text input has a valid email format in ReactJS?
Solution 1
Everybody made some valid points here, And you will have to follow almost all of these to validate the data.
Anyways, As of now i think this would be better way to move forward with email validation.
- Set
<input type="email" />
so browsers can validate the email for you - Validate it using javascript
- Perform validation on server
You can render the input like
<input type="email" value={ this.state.email } onChange={ handleOnChange } />
And your handleOnChange
function code will look something like this
let handleOnChange = ( email ) => {
// don't remember from where i copied this code, but this works.
let re = /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
if ( re.test(email) ) {
// this is a valid email address
// call setState({email: email}) to update the email
// or update the data in redux store.
}
else {
// invalid email, maybe show an error to the user.
}
}
NOTE : Depending on how you've setup everything you might have to use this.handleOnChange
instead of handleOnChange
and also bind it in constructor
like this.handleOnChange = this.handleOnChange.bind(this);
...
And when you make post request to the server/api with the email address
, Validate the email on the server and if valid store it in database otherwise respond back with error.
You can just google node* validation libraries and use them to handle all the validations, you could use the email validation code from here... or validate it however you want.
Solution 2
It can be performed using javascript + regular expression validation
You can disable the submit button or else add a text warning till the email input matches the regEx format .
HTML:
<input type="text" name="email" id="email" placeholder="Email" onKeyPress="emailVerify(this.id)">
JAVASCRIPT:
function emailVerify(x){
var testEmail = /^[ ]*([^@\s]+)@((?:[-a-z0-9]+\.)+[a-z]{2,})[ ]*$/i;
jQuery('input').bind('input propertychange', function() {
if (testEmail.test(jQuery(this).val()))
{
// action to be performed if email is valid
}
else
{
// action to be performed if email is invalid - like disabling the submit button
}
});
}
The function emailVerify()
should be invkoed during every "key press" inside the email text box.
The function .bind()
recrod all the key down event i.e. whenever you press the key it will check whether your email text box input matched the regular expression (testEmail charecters)
An email of the form [email protected] will be considered whereas an email me/@me.co will be considered invalid
Solution 3
It depends on how your TextField component is implemented, but typically with React you get the text input field value via the onChange event and put it in the component state or somewhere else (like your redux store). Your code may look something like this:
<input
type="text"
value={this.state.email}
onChange={event => this.setState({email: event.target.value})
/>
Now you can simply check for the validity of the email in your onChange handler, but that might be too expensive (because you essentially re-check at every key press). Another solution would be to do the check when the component loses focus, like so:
<input
type="text"
value={this.state.email}
onChange={event => this.setState({email: event.target.value})
onBlur={() => this.setState({
emailIsValid: isValidEmailAddress(this.state.email)
})
/>
And then you can get feedback to users according to the value of the emailIsValid boolean in your component state, for example with a css class.
EDIT: Salehen Rahman made a valid point about the type="email" option. The paragraph below is maybe not that relevant.
As for the isValidEmailAddress implementation, a regular expression is a reasonable choice. There are more or less complex regular expressions to determine valid emails but just having a non-empty string, then @, then another non-empty string is a good approximation. So something like that could do:
function isValidEmailAddress(address) {
return !! address.match(/.+@.+/);
}
Solution 4
Form validation should be done on both sides: client side and server side as well, here is a good example to understand how to implement form validations in React, using Flux architecture
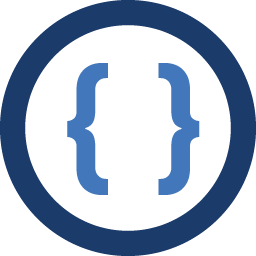
Admin
Updated on July 13, 2022Comments
-
Admin almost 2 years
In ReactJS, what's the correct way to verify if an entered text (
<TextField/>
) is in email format, such as with @?I have the application connected to MongoDB, so should I be doing the email format check on the client-side or on server-side?
Any example or guidance would be greatly appreciated.
Thank you in advance!
-
Sal Rahman over 7 yearsDepending on the browser, email checks do not even need any RegExp checks. Just set the
type
to"email"
, and the browser will warn the user that the entered string is not an email address. You only perform email checks for those dinosaur-aged browsers that don't have"email"
as an input type. And also, perhaps consider using a library for email validation. For JavaScript we have validator.js: github.com/chriso/validator.js