how to check if SharedPreferences exists for this application in flutter
Solution 1
You need to check if the key exists yet by using prefs.containsKey('username')
.
Solution 2
When I use "shared_preferences", I personally use an helper to get and set just like this :
GETTER
static Future<String> getUserName() async {
final SharedPreferences prefs = await SharedPreferences.getInstance();
return prefs.getString('username') ?? '';
}
SETTER
static Future<String> setUserName(String value) async {
final SharedPreferences prefs = await SharedPreferences.getInstance();
return prefs.setString('username', value);
}
To answer your question, you can't check if the SharedPreferences "file" exist or not. Your method is already correct.
Solution 3
Though already you got your answer, nevertheless here I mentioned how I used in my app.
Set the value using this
SharedPreferences prefs = await SharedPreferences.getInstance();
prefs.setBool("login", true);
Check by containsKey in Login page
SharedPreferences prefs = await SharedPreferences.getInstance();
if (!prefs.containsKey("login")) {
Navigator.of(context).push(MaterialPageRoute(
builder: (context) {
return LoginPage();
},
));
}
also check in logout page like this
SharedPreferences prefs = await SharedPreferences.getInstance();
if(prefs.containsKey("login")) {
prefs.remove("login");
Utils.successToast("Logout successfully");
Navigator.of(context).pop();
}
Note : you can use whatever you want like 'username' or something else instead on "login"
Solution 4
You are getting empty because you do not have your SharedPreferences KEY yet;
so just put ??
operator and return a value.
// Separated shared preferences method is a good call
Future<bool> isUserLoggedIn() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
return prefs.getBool(IS_LOGGED_KEY) ?? false;
}
// Your logic
void checkingTheSavedData() {
isUserLoggedIn().then((isLogged) {
if (isLogged) {
// foo
} else {
// bar
}
});
}
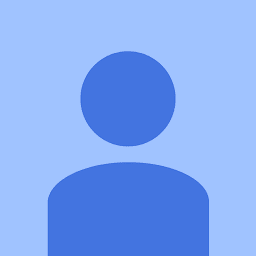
Comments
-
Ahmed Wagdi over 1 year
my flutter application checks for saved data in
SharedPreferences
every time it starts . to use it later in the application, but when I run the app for the first time in a device, there is savedSharedPreferences
yet so it gets all the data asnull
. so I want to make sure that it checks if the file itself exists rather than checking for a specific value,note: I'm using this shared_preferences futter package.
here is my code :
checkingTheSavedData() async { SharedPreferences prefs = await SharedPreferences.getInstance(); String username = prefs.getString('username'); if (username == null) { Navigator.pushReplacementNamed(context, '/login'); } else { Navigator.pushReplacementNamed(context, '/main_page'); } }
So, for the frist run it does
Navigator.pushReplacementNamed(context, '/main_page');
but with empty data , after that when i do a real login and the data is already saved , it works fine. any idea how to handle this in a proper way ?