How to clone and integrate external (from git) cmake project into local one
I would go with the first approach. You don't need to specify a build command because cmake is used by default. This could look like:
cmake_minimum_required(VERSION 3.0)
project(GTestProject)
include(ExternalProject)
set(EXTERNAL_INSTALL_LOCATION ${CMAKE_BINARY_DIR}/external)
ExternalProject_Add(googletest
GIT_REPOSITORY https://github.com/google/googletest
CMAKE_ARGS -DCMAKE_INSTALL_PREFIX=${EXTERNAL_INSTALL_LOCATION}
)
include_directories(${EXTERNAL_INSTALL_LOCATION}/include)
link_directories(${EXTERNAL_INSTALL_LOCATION}/lib)
add_executable(FirstTest main.cpp)
add_dependencies(FirstTest googletest)
target_link_libraries(FirstTest gtest gtest_main pthread)
I don't know if this is the correct/preferred way if there even is one. If you wanted to implement your second approach you would have to download the code with execute_process first.
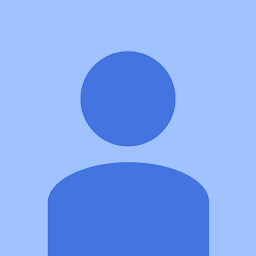
amigo421
Updated on July 05, 2022Comments
-
amigo421 almost 2 years
I faced with a problem when I was trying to use Google Test.
There are lot of manuals on how to use
ExternalProject_Add
for the adding gtest into the project, however most of these describe a method based on downloading zip archive with gtest and build it.As we know gtest is github-hosted and cmake-based project. So I'd like to find native cmake way.
If this would be a header-only project, I'd write something like:
cmake_minimum_required(VERSION 2.8.8) include(ExternalProject) find_package(Git REQUIRED) ExternalProject_Add( gtest PREFIX ${CMAKE_CURRENT_SOURCE_DIR}/ext GIT_REPOSITORY https://github.com/google/googletest.git TIMEOUT 10 UPDATE_COMMAND ${GIT_EXECUTABLE} pull CONFIGURE_COMMAND "" BUILD_COMMAND "" INSTALL_COMMAND "" LOG_DOWNLOAD ON ) ExternalProject_Get_Property(gtest source_dir) set(GTEST_INCLUDE_DIR ${source_dir}/googletest/include CACHE INTERNAL "Path to include folder for GTest") set(GTEST_ROOT_DIR ${source_dir}/googletest CACHE INTERNAL "Path to source folder for GTest") include_directories(${INCLUDE_DIRECTORIES} ${GTEST_INCLUDE_DIR} ${GTEST_ROOT_DIR}) message(${GTEST_INCLUDE_DIR})
and add this script from my cmake project like:
set(CMAKE_MODULE_PATH ${CMAKE_MODULE_PATH} "${CMAKE_SOURCE_DIR}/cmake.modules/") include(AddGTest) .... add_dependencies(${PROJECT_NAME} gtest)
However this requires a build step.
How should this be implemented?
- By adding
BUILD_COMMAND
intoExternaProject_Add
and linking with produced libs? - Or by including gtest's cmakelists into my project, something like this:
add_subdirectory (${CMAKE_SOURCE_DIR}\ext\src\gtest\googletest\CMakeLists.txt)
this is not correct way because on the moment of the project load the folder does not exist.
- Any other ways?
What is a correct/prefer way?
- By adding
-
amigo421 almost 8 yearsThank you, this is what i want to hear - "cmake does this by default" , didn't find this in manual, and looked for build command
-
Craig Scott almost 6 yearsThis article documents a way that the gtest manual now also recommends. CMake 3.11 added the FetchContent module which extends the approach further and uses gtest as one of its examples.
-
ataraxis over 3 years@CraigScott Your answers are always the most enlightening ones. This is worth a own answer instead of just a comment. I really love your book by the way!