How to compare string to enum type in Java?
52,760
Solution 1
try this
public void setState(String s){
state = State.valueOf(s);
}
You might want to handle the IllegalArgumentException that may be thrown if "s" value doesn't match any "State"
Solution 2
Use .name()
method. Like st.name()
. e.g. State.AL.name()
returns string "AL".
So,
if(st.name().equalsIgnoreCase(s)) {
should work.
Solution 3
to compare enum to string
for (Object s : State.values())
{
if (theString.equals(s.toString()))
{
// theString is equal State object
}
}
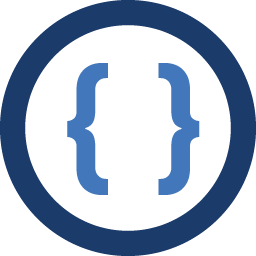
Author by
Admin
Updated on October 03, 2020Comments
-
Admin over 3 years
I have an enum list of all the states in the US as following:
public enum State { AL, AK, AZ, AR, ..., WY }
and in my test file, I will read input from a text file that contain the state. Since they are string, how can I compare it to the value of enum list in order to assign value to the variable that I have set up as:
private State state;
I understand that I need to go through the enum list. However, since the values are not string type, how can you compare it? This is what I just type out blindly. I don't know if it's correct or not.
public void setState(String s) { for (State st : State.values()) { if (s == State.values().toString()) { s = State.valueOf(); break; } } }
-
Luiggi Mendoza over 11 yearsIt should handle the
IllegalArgumentException
though. -
Matt over 11 years.toString() is not really the right way to do it, though it will work. The .name() method is preferred as you can override the .toString() method to do anything you want in an enum class, but the .name() method is declared as final in the Enum class (which is the parent class of any java enum).