How to compile C and Gtk+ with GCC on Linux?
Solution 1
For starters, you write your C code, say "hello_world_gtk.c", and then you compile and link it against Gtk, by using appropriate compiler and linker flags. These flags are given to you by the pkg-config tool. To get the needed compiler flags, you call that tool with:
pkg-config gtk+-2.0 --cflags
For the link flags:
pkg-config gtk+-2.0 --libs
The backticks allow you to get the output from pkg-config and pass it on as arguments to gcc:
gcc `pkg-config gtk+-2.0 --cflags` hello_world_gtk.c -o hello_world_gtk `pkg-config gtk+-2.0 --libs`
But you're not required to use backticks. You can just copy&paste the flags manually if you want.
For Gtk 3, replace "2.0" with "3.0". If pkg-config reports that it couldn't find the package, then you didn't install the Gtk development package as offered by your Linux distribution.
If you generally don't understand how you compile something into object files and then link it, then you shouldn't begin with Gtk, but with plain C instead. Once you're familiar with the absolute basics of compiling and linking, then you can move on to Gtk applications.
Solution 2
by gtk2.0 example:
$gcc test.c -o gnometest $(pkg-config --cflags --libs gtk+-2.0)
by gtk3.0 example:
$gcc $(pkg-config --cflags gtk+-3.) test.c -o gnometest $(pkg-config --libs gtk+-3.0)
Solution 3
From the Gtk Docs Getting Started page:
gcc `pkg-config --cflags gtk+-3.0` -o example example.c `pkg-config --libs gtk+-3.0`
Also check Nikos C.'s Aswer to learn more about pkg-config
.
Solution 4
As others said, your question is overly broad. It asks about backticks (basic shell syntax), what ".o" files are (basic programming), and GTK programming (somewhat advanced programming).
But to answer it best I can, and assuming you just have a single source file, use
gcc -Wall -Wextra -std=c99 `pkg-config --cflags --libs gtk+-2.0` example.c -o example
where "example.c" is the name of your source. Here's the breakdown:
Use the flags "-Wall" and "-Wextra" so you can see all the warnings (and fix them).
Use the "std" flag to set your C dialect. There are several and gcc has either total or partial support for most of them. Read the man pages or info pages for more information. Using "c99" like above is not a bad choice.
And use pkg-config and command substitution (i.e., backticks) to set the libraries and includes. Notice you can do both at once, although a lot of people use it twice by two backticked versions of pkg-config. Backticks substitute the output from the command within the ticks. Try running the pkg-config within the backquotes by itself on the command line and you'll see what it does.
Unfortunately, there aren't many resources for 3.x GTK code and most of it is still 2.x based. You'll just have to resort to either gtk mailing lists or the reference manual.
As for GTK 3.x, the reason you can't compile for it is likely that your distribution is GTK 2.x based. Newer distributions may have GTK 3.x in their repos.
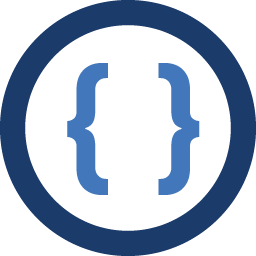
Admin
Updated on July 12, 2022Comments
-
Admin almost 2 years
I've searched and searched but I'm not getting the information I really want. Can someone please explain, as completely and fundamentally as possible, how Gtk+ code is compiled when writing in C, using GCC, on Linux. There's things like backticks, "c99", and .o files that I don't understand at all.
I'd also appreciate any resources for learning Gtk+ code. All the sources I've found are for versions 2.x, but I think 3.6 is the current version.
I'd like to reiterate, I'm only interested in C code. Please don't try explaining to me the benefits of C++ or C#, I've read all of them. I'm here for C. Thank you!
-
Admin about 11 yearsI got it compiled and running, Something was wrong with my installation. Although I was only able to get 2.0 installed, not any 3.x version.
-
petermeissner over 8 yearsnice, works for gtk 3.0 as well: LDLIBS = -lcurl -lpthread
pkg-config --cflags gtk+-3.0
pkg-config --libs gtk+-3.0
-
ThorSummoner about 4 yearsi would also gently encourage the preference of
$(subcommand)
instead of`subcommand`
, the former can be nested directly while the latter is likely to do nearly undefined things when nested without care -
ThorSummoner about 4 yearsor Equivalently, using GNU Make and its environs,
env CFLAGS="$(pkg-config --cflags gtk+-3.0)" LDLIBS="$(pkg-config --libs gtk+-3.0)" make example