How to connect UCanAccess to an Access database encrypted with a database password?
Steps of How to connect UCanAccess to an Access database encrypted with a database password
Step 1:
Add these two packages to your project (jackcess-encrypt.jar, bcprov-ext-jdk15on-152)
You can download the two packages from the following links:
Jackcess Encrypt
Bouncy Castle
Step 2:
You have to add this class to your project folder
import java.io.File;
import java.io.IOException;
import net.ucanaccess.jdbc.JackcessOpenerInterface;
import com.healthmarketscience.jackcess.CryptCodecProvider;
import com.healthmarketscience.jackcess.Database;
import com.healthmarketscience.jackcess.DatabaseBuilder;
public class CryptCodecOpener implements JackcessOpenerInterface {
@Override
public Database open(File fl,String pwd) throws IOException {
DatabaseBuilder dbd =new DatabaseBuilder(fl);
dbd.setAutoSync(false);
dbd.setCodecProvider(new CryptCodecProvider(pwd));
dbd.setReadOnly(false);
return dbd.open();
}
//Notice that the parameter setting autosync =true is recommended with UCanAccess for performance reasons.
//UCanAccess flushes the updates to disk at transaction end.
//For more details about autosync parameter (and related tradeoff), see the Jackcess documentation.
}
like this
step 3:
use the following connection code
public void connectToDB(){
try {
conn = DriverManager.getConnection("jdbc:ucanaccess://words.accdb;jackcessOpener=CryptCodecOpener", "user", "pass");
} catch (SQLException ex) {
ex.printStackTrace();
}
}
You can also watch this video...https://www.youtube.com/watch?v=TT6MgBBkRSE
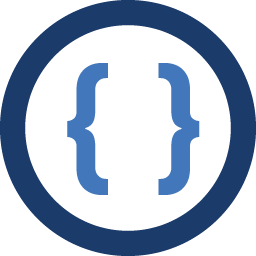
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I've developed a Java application (a dictionary) with an Access database to store the words of the dictionary and I'm getting ready to distribute it. I want to encrypt my database with a password to prevent people to access my words. When I set a passwords the Java code shows this Exception
net.ucanaccess.jdbc.UcanaccessSQLException: Decoding not supported. Please choose a CodecProvider which supports reading the current database encoding. at net.ucanaccess.jdbc.UcanaccessDriver.connect(UcanaccessDriver.java:247)
Here is my connection code before encryption of my database with password ....
String s1="jdbc:ucanaccess://"; String user=""; String pass=""; String s4="words.accdb"; public void connectToDB(){ //database connection try { conn = DriverManager.getConnection(s1+s4,user,pass); } catch (SQLException e) { e.printStackTrace(); } //end of database connection }
Here is the code after the encryption with password for example 12345...
String s1="jdbc:ucanaccess://"; String user=""; String pass="12345"; String s4="words.accdb"; public void connectToDB(){ //database connection try { conn = DriverManager.getConnection(s1+s4,user,pass); } catch (SQLException e) { e.printStackTrace(); } //end of database connection }